
- PHP All Exercises & Assignments
Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database.
Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.

Write a program to count 5 to 15 using PHP loop
Description: Write a Program to display count, from 5 to 15 using PHP loop as given below.
Rules & Hint
- You can use “for” or “while” loop
- You can use variable to initialize count
- You can use html tag for line break
View Solution/Program
5 6 7 8 9 10 11 12 13 14 15
Write a program to print “Hello World” using echo
Description: Write a program to print “Hello World” using echo only?
Conditions:
- You can not use any variable.
View Solution /Program
Hello World
Write a program to print “Hello PHP” using variable
Description: Write a program to print “Hello PHP” using php variable?
- You can not use text directly in echo but can use variable.
Write a program to print a string using echo+variable.
Description: Write a program to print “Welcome to the PHP World” using some part of the text in variable & some part directly in echo.
- You have to use a variable that contains string “PHP World”.
Welcome to the PHP World
Write a program to print two variables in single echo
Description: Write a program to print 2 php variables using single echo statement.
- First variable have text “Good Morning.”
- Second variable have text “Have a nice day!”
- Your output should be “Good morning. Have a nice day!”
- You are allowed to use only one echo statement in this program.
Good Morning. Have a nice day!
Write a program to check student grade based on marks
Description:.
Write a program to check student grade based on the marks using if-else statement.
- If marks are 60% or more, grade will be First Division.
- If marks between 45% to 59%, grade will be Second Division.
- If marks between 33% to 44%, grade will be Third Division.
- If marks are less than 33%, student will be Fail.
Click to View Solution/Program
Third Division
Write a program to show day of the week using switch
Write a program to show day of the week (for example: Monday) based on numbers using switch/case statements.
- You can pass 1 to 7 number in switch
- Day 1 will be considered as Monday
- If number is not between 1 to 7, show invalid number in default
It is Friday!
Write a factorial program using for loop in php
Write a program to calculate factorial of a number using for loop in php.
The factorial of 3 is 6
Factorial program in PHP using recursive function
Exercise Description: Write a PHP program to find factorial of a number using recursive function .
What is Recursive Function?
- A recursive function is a function that calls itself.
Write a program to create Chess board in PHP using for loop
Write a PHP program using nested for loop that creates a chess board.
- You can use html table having width=”400px” and take “30px” as cell height and width for check boxes.

Write a Program to create given pattern with * using for loop
Description: Write a Program to create following pattern using for loops:
- You can use for or while loop
- You can use multiple (nested) loop to draw above pattern
View Solution/Program using two for loops
* ** *** **** ***** ****** ******* ********
Simple Tips for PHP Beginners
When a beginner start PHP programming, he often gets some syntax errors. Sometimes these are small errors but takes a lot of time to fix. This happens when we are not familiar with the basic syntax and do small mistakes in programs. These mistakes can be avoided if you practice more and taking care of small things.
I would like to say that it is never a good idea to become smart and start copying. This will save your time but you would not be able to understand PHP syntax. Rather, Type your program and get friendly with PHP code.
Follow Simple Tips for PHP Beginners to avoid errors in Programming
- Start with simple & small programs.
- Type your PHP program code manually. Do not just Copy Paste.
- Always create a new file for new code and keep backup of old files. This will make it easy to find old programs when needed.
- Keep your PHP files in organized folders rather than keeping all files in same folder.
- Use meaningful names for PHP files or folders. Some examples are: “ variable-test.php “, “ loops.php ” etc. Do not just use “ abc.php “, “ 123.php ” or “ sample.php “
- Avoid space between file or folder names. Use hyphens (-) instead.
- Use lower case letters for file or folder names. This will help you make a consistent code
These points are not mandatory but they help you to make consistent and understandable code. Once you practice this for 20 to 30 PHP programs, you can go further with more standards.
The PHP Standard Recommendation (PSR) is a PHP specification published by the PHP Framework Interop Group.
Experiment with Basic PHP Syntax Errors
When you start PHP Programming, you may face some programming errors. These errors stops your program execution. Sometimes you quickly find your solutions while sometimes it may take long time even if there is small mistake. It is important to get familiar with Basic PHP Syntax Errors
Basic Syntax errors occurs when we do not write PHP Code correctly. We cannot avoid all those errors but we can learn from them.
Here is a working PHP Code example to output a simple line.
Output: Hello World!
It is better to experiment with PHP Basic code and see what errors happens.
- Remove semicolon from the end of second line and see what error occurs
- Remove double quote from “Hello World!” what error occurs
- Remove PHP ending statement “?>” error occurs
- Use “
- Try some space between “
Try above changes one at a time and see error. Observe What you did and what error happens.
Take care of the line number mentioned in error message. It will give you hint about the place where there is some mistake in the code.
Read Carefully Error message. Once you will understand the meaning of these basic error messages, you will be able to fix them later on easily.
Note: Most of the time error can be found in previous line instead of actual mentioned line. For example: If your program miss semicolon in line number 6, it will show error in line number 7.
Using phpinfo() – Display PHP Configuration & Modules
phpinfo() is a PHP built-in function used to display information about PHP’s configuration settings and modules.
When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality. For example, the date-time extension provides some ready-made function related to date and time formatting. MySQL modules are integrated to deal with PHP Connections.
It is good to take a look on those extensions. Simply use
phpinfo() function as given below.
Example Using phpinfo() function

Write a PHP program to add two numbers
Write a program to perform sum or addition of two numbers in PHP programming. You can use PHP Variables and Operators
PHP Program to add two numbers:
Write a program to calculate electricity bill in php.
You need to write a PHP program to calculate electricity bill using if-else conditions.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements .
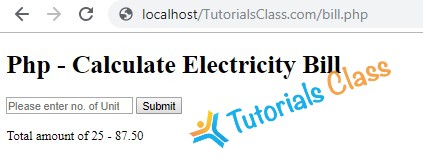
Write a simple calculator program in PHP using switch case
You need to write a simple calculator program in PHP using switch case.
Operations:
- Subtraction
- Multiplication

Remove specific element by value from an array in PHP?
You need to write a program in PHP to remove specific element by value from an array using PHP program.
Instructions:
- Take an array with list of month names.
- Take a variable with the name of value to be deleted.
- You can use PHP array functions or foreach loop.
Solution 1: Using array_search()
With the help of array_search() function, we can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 2: Using foreach()
By using foreach() loop, we can also remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 3: Using array_diff()
With the help of array_diff() function, we also can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [2]=> string(5) “march” [4]=> string(3) “may” }
Write a PHP program to check if a person is eligible to vote
Write a PHP program to check if a person is eligible to vote or not.
- Minimum age required for vote is 18.
- You can use PHP Functions .
- You can use Decision Making Statements .
Click to View Solution/Program.
You Are Eligible For Vote
Write a PHP program to calculate area of rectangle
Write a PHP program to calculate area of rectangle by using PHP Function.
- You must use a PHP Function .
- There should be two arguments i.e. length & width.
View Solution/Program.
Area Of Rectangle with length 2 & width 4 is 8 .
- Next »
- PHP Exercises Categories
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
- Language Reference
Assignment Operators
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The value of an assignment expression is the value assigned. That is, the value of " $a = 3 " is 3. This allows you to do some tricky things: <?php $a = ( $b = 4 ) + 5 ; // $a is equal to 9 now, and $b has been set to 4. ?>
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic , array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression. For example: <?php $a = 3 ; $a += 5 ; // sets $a to 8, as if we had said: $a = $a + 5; $b = "Hello " ; $b .= "There!" ; // sets $b to "Hello There!", just like $b = $b . "There!"; ?>
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. This may also have relevance if you need to copy something like a large array inside a tight loop.
An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Assignment by Reference
Assignment by reference is also supported, using the " $var = &$othervar; " syntax. Assignment by reference means that both variables end up pointing at the same data, and nothing is copied anywhere.
Example #1 Assigning by reference
The new operator returns a reference automatically, as such assigning the result of new by reference is an error.
The above example will output:
More information on references and their potential uses can be found in the References Explained section of the manual.
Arithmetic Assignment Operators
Bitwise assignment operators, other assignment operators.
- arithmetic operators
- bitwise operators
- null coalescing operator
Improve This Page
User contributed notes.

PHP Tutorial
Php advanced, mysql database, php examples, php reference, php operators.
Operators are used to perform operations on variables and values.
PHP divides the operators in the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators

PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Advertisement
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
The PHP decrement operators are used to decrement a variable's value.
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
PHP String Operators
PHP has two operators that are specially designed for strings.
PHP Array Operators
The PHP array operators are used to compare arrays.
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
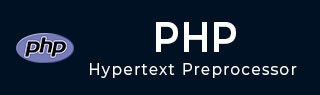
- PHP Tutorial
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Cheatsheet
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Online Compiler
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP - Assignment Operators Examples
You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the "=" operator assigns the value on the right-hand side to the variable on the left-hand side.
Additionally, there are compound assignment operators like +=, -= , *=, /=, and %= which combine arithmetic operations with assignment. For example, "$x += 5" is a shorthand for "$x = $x + 5", incrementing the value of $x by 5. Assignment operators offer a concise way to update variables based on their current values.
The following table highligts the assignment operators that are supported by PHP −
The following example shows how you can use these assignment operators in PHP −
It will produce the following output −
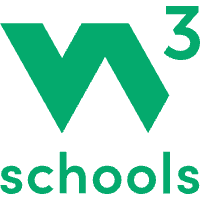
PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP Assignment Operators: A Comprehensive Guide for Beginners
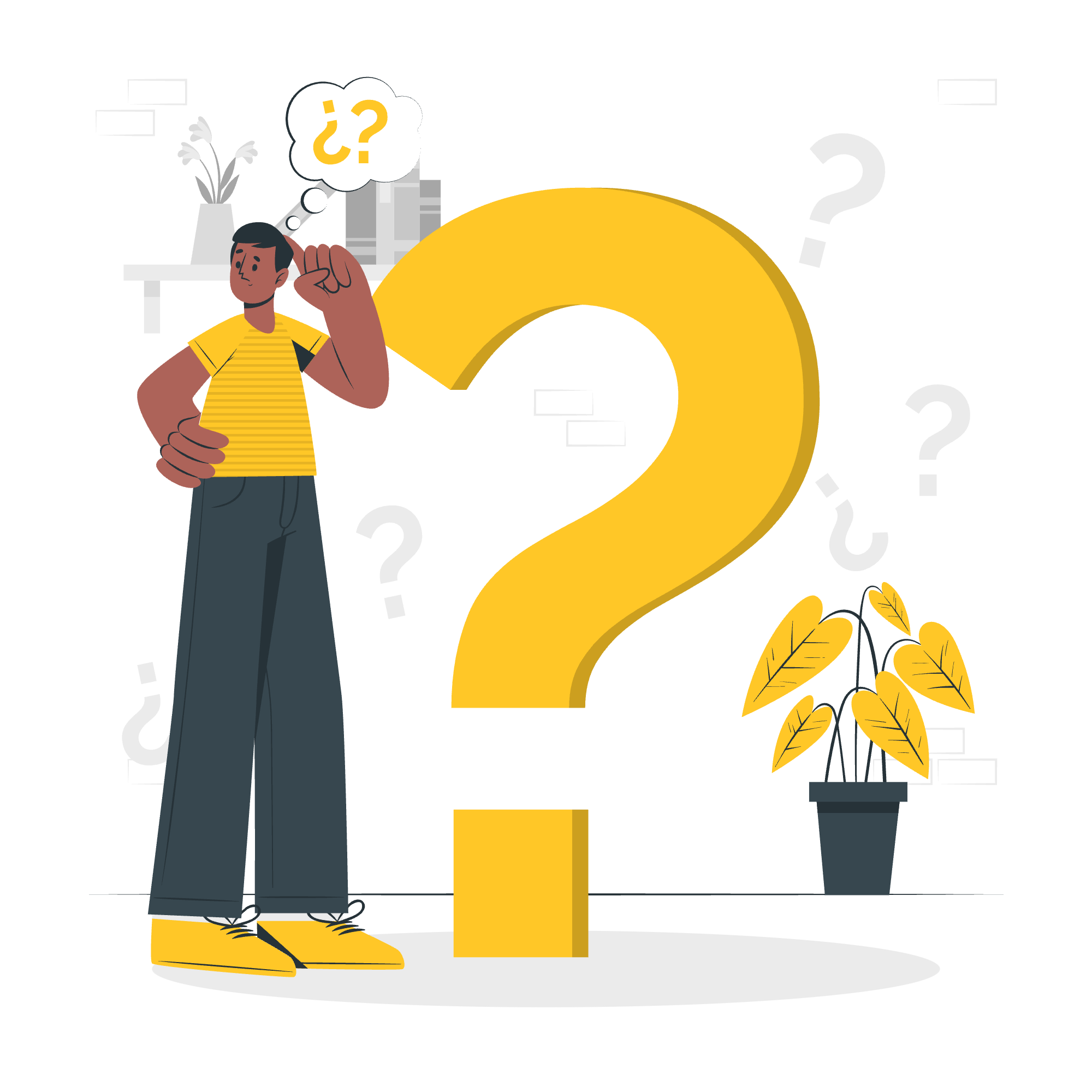
What Are Assignment Operators?
Before we dive into the deep end, let's start with the basics. Assignment operators in PHP are like the helpful assistants in your code kitchen. They take a value and assign it to a variable, much like you'd put ingredients into a mixing bowl. The most common assignment operator is the humble equals sign (=).
The Basic Assignment Operator (=)
Let's look at a simple example:
In this case, we're telling PHP, "Hey, please remember that my_age is 25." It's that simple!
Compound Assignment Operators
Now, let's spice things up a bit. PHP has a set of compound assignment operators that combine an arithmetic operation with assignment. These operators are like kitchen gadgets that chop and mix at the same time – they're real time-savers!
Here's a table of the compound assignment operators in PHP:
Let's break these down with some tasty examples!
The Addition Assignment Operator (+=)
Here, we started with 5 apples and added 3 more. The += operator does this in one swift motion!
The Subtraction Assignment Operator (-=)
Oops! Someone ate 2 cookies. The -= operator helps us keep track of our dwindling cookie supply.
The Multiplication Assignment Operator (*=)
Rabbits multiply fast, don't they? The *= operator helps us model this rapid growth!
The Division Assignment Operator (/=)
Sharing is caring! We've divided our pizza slices by 2, perhaps to share with a friend.
The Modulus Assignment Operator (%=)
This one's a bit tricky. It divides $candies by 5 and gives us the remainder. It's like asking, "If we share 17 candies among 5 people, how many are left over?"
The Exponentiation Assignment Operator (**=)
Bacteria can double every generation. This operator helps us calculate exponential growth easily!
String Concatenation Assignment Operator (.=)
Last but not least, let's talk about the string concatenation assignment operator. It's like a word game where we keep adding new words to make a sentence.
We started with "Hello" and added " World" to it. The .= operator does this joining for us in one step!
Practical Examples
Now that we've covered all the operators, let's put them to use in a fun little program:
This program simulates a simple game where a player's score changes based on various events. Each line demonstrates a different assignment operator in action.
And there you have it, folks! We've journeyed through the land of PHP assignment operators, from the simple = to the more complex compound operators. Remember, these operators are your friends – they're here to make your coding life easier and your programs more efficient.
Practice using these operators in your own code. Try creating a simple calculator or a text-based game. The more you use them, the more natural they'll become.
Happy coding, and may your variables always be correctly assigned!
Credits: Image by storyset
PHP Assignment Operators
Php tutorial index.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
- Language Reference
- Control Structures
Introduction
Any PHP script is built out of a series of statements. A statement can be an assignment, a function call, a loop, a conditional statement or even a statement that does nothing (an empty statement). Statements usually end with a semicolon. In addition, statements can be grouped into a statement-group by encapsulating a group of statements with curly braces. A statement-group is a statement by itself as well. The various statement types are described in this chapter.
The following are also considered language constructs even though they are referenced under functions in the manual.
Found A Problem?
User contributed notes.


IMAGES
VIDEO
COMMENTS
PHP is a popular general-purpose scripting language that powers everything from your blog to the most popular websites in the world. ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
But mixing logical and assignment operators in one statement is bad. This is called obfuscation and perl write-only style, makes reading this code harder. So, it's better to be written as ... One PHP statement to use result of IF statement for assignment. 6. PHP: Assign multiple variables in if statement. 1.
phpinfo() is a PHP built-in function used to display information about PHP's configuration settings and modules. When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality.
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right. ... The PHP logical operators are used to combine conditional statements. Operator Name Example Result Try it; and ...
Use PHP assignment operator (=) to assign a value to a variable. The assignment expression returns the value assigned. ... (.=)to concatenate strings and assign the result to a variable in a single statement. Did you find this tutorial useful? Yes No . Send Cancel. Previously. PHP Operators. Up Next. PHP Comparison Operators. Search for ...
PHP - Assignment Operators Examples - You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the = operator assigns the value on the right-hand side to the variable on the left-han
Compound Assignment Operators. Now, let's spice things up a bit. PHP has a set of compound assignment operators that combine an arithmetic operation with assignment. These operators are like kitchen gadgets that chop and mix at the same time - they're real time-savers! Here's a table of the compound assignment operators in PHP:
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
PHP is a popular general-purpose scripting language that powers everything from your blog to the most popular websites in the world. ... Any PHP script is built out of a series of statements. A statement can be an assignment, a function call, a loop, a conditional statement or even a statement that does nothing (an empty statement). ...