

25+ JavaScript Coding Interview Questions (SOLVED with CODE)
Having a JavaScript Coding Interview Session on this week? Fear not, we got your covered! Check that ultimate list of 25 advanced and tricky JavaScript Coding Interview Questions and Challenges to crack on your next senior web developer interview and got your next six-figure job offer in no time!
Q1 : Explain what a callback function is and provide a simple example
A callback function is a function that is passed to another function as an argument and is executed after some operation has been completed. Below is an example of a simple callback function that logs to the console after some operations have been completed.
Q2 : Given a string, reverse each word in the sentence
For example Welcome to this Javascript Guide! should be become emocleW ot siht tpircsavaJ !ediuG
Q3 : How to check if an object is an array or not? Provide some code.
The best way to find whether an object is instance of a particular class or not using toString method from Object.prototype
One of the best use cases of type checking of an object is when we do method overloading in JavaScript. For understanding this let say we have a method called greet which take one single string and also a list of string, so making our greet method workable in both situation we need to know what kind of parameter is being passed, is it single value or list of value?
However, in above implementation it might not necessary to check type for array, we can check for single value string and put array logic code in else block, let see below code for the same.
Now it's fine we can go with above two implementations, but when we have a situation like a parameter can be single value , array , and object type then we will be in trouble.
Coming back to checking type of object, As we mentioned that we can use Object.prototype.toString
If you are using jQuery then you can also used jQuery isArray method:
FYI jQuery uses Object.prototype.toString.call internally to check whether an object is an array or not.
In modern browser, you can also use:
Array.isArray is supported by Chrome 5, Firefox 4.0, IE 9, Opera 10.5 and Safari 5
Q4 : How to empty an array in JavaScript?
How could we empty the array above?
Above code will set the variable arrayList to a new empty array. This is recommended if you don't have references to the original array arrayList anywhere else because It will actually create a new empty array. You should be careful with this way of empty the array, because if you have referenced this array from another variable, then the original reference array will remain unchanged, Only use this way if you have only referenced the array by its original variable arrayList .
For Instance:
Above code will clear the existing array by setting its length to 0. This way of empty the array also update all the reference variable which pointing to the original array. This way of empty the array is useful when you want to update all the another reference variable which pointing to arrayList .
Above implementation will also work perfectly. This way of empty the array will also update all the references of the original array.
Above implementation can also empty the array. But not recommended to use often.
Q5 : How would you check if a number is an integer?
A very simply way to check if a number is a decimal or integer is to see if there is a remainder left when you divide by 1.
Q6 : Implement enqueue and dequeue using only two stacks
Enqueue means to add an element, dequeue to remove an element.
Q7 : Make this work
Q8 : write a "mul" function which will properly when invoked as below syntax.
Here mul function accept the first argument and return anonymous function which take the second parameter and return anonymous function which take the third parameter and return multiplication of arguments which is being passed in successive
In JavaScript function defined inside has access to outer function variable and function is the first class object so it can be returned by function as well and passed as argument in another function.
- A function is an instance of the Object type
- A function can have properties and has a link back to its constructor method
- Function can be stored as variable
- Function can be pass as a parameter to another function
- Function can be returned from function
Q9 : Write a function that would allow you to do this?
You can create a closure to keep the value passed to the function createBase even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable baseNumber .
Q10 : FizzBuzz Challenge
Create a for loop that iterates up to 100 while outputting "fizz" at multiples of 3 , "buzz" at multiples of 5 and "fizzbuzz" at multiples of 3 and 5 .
Check out this version of FizzBuzz:
Q11 : Given two strings, return true if they are anagrams of one another
For example: Mary is an anagram of Army
Q12 : How would you use a closure to create a private counter?
You can create a function within an outer function (a closure) that allows you to update a private variable but the variable wouldn't be accessible from outside the function without the use of a helper function.
Q13 : Provide some examples of non-bulean value coercion to a boolean one
The question is when a non-boolean value is coerced to a boolean, does it become true or false , respectively?
The specific list of "falsy" values in JavaScript is as follows:
- "" (empty string)
- 0 , -0 , NaN (invalid number)
- null , undefined
Any value that's not on this "falsy" list is "truthy." Here are some examples of those:
- [ ] , [ 1, "2", 3 ] (arrays)
- { } , { a: 42 } (objects)
- function foo() { .. } (functions)
Q14 : What will be the output of the following code?
Above code would give output 1undefined . If condition statement evaluate using eval so eval(function f() {}) which return function f() {} which is true so inside if statement code execute. typeof f return undefined because if statement code execute at run time, so statement inside if condition evaluated at run time.
Above code will also output 1undefined .
Q15 : What will the following code output?
The code above will output 5 even though it seems as if the variable was declared within a function and can't be accessed outside of it. This is because
is interpreted the following way:
But b is not declared anywhere in the function with var so it is set equal to 5 in the global scope .
Q16 : Write a function that would allow you to do this
You can create a closure to keep the value of a even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable a .
Q17 : How does the this keyword work? Provide some code examples
In JavaScript this always refers to the “owner” of the function we're executing, or rather, to the object that a function is a method of.
Q18 : How would you create a private variable in JavaScript?
To create a private variable in JavaScript that cannot be changed you need to create it as a local variable within a function. Even if the function is executed the variable cannot be accessed outside of the function. For example:
To access the variable, a helper function would need to be created that returns the private variable.
Q19 : What is Closure in JavaScript? Provide an example
A closure is a function defined inside another function (called parent function) and has access to the variable which is declared and defined in parent function scope.
The closure has access to variable in three scopes:
- Variable declared in his own scope
- Variable declared in parent function scope
- Variable declared in global namespace
innerFunction is closure which is defined inside outerFunction and has access to all variable which is declared and defined in outerFunction scope. In addition to this function defined inside function as closure has access to variable which is declared in global namespace .
Output of above code would be:
Q20 : What will be the output of the following code?
Above code will output 0 as output. delete operator is used to delete a property from an object. Here x is not an object it's local variable . delete operator doesn't affect local variable.
Q21 : What will be the output of the following code?
Above code will output xyz as output. Here emp1 object got company as prototype property. delete operator doesn't delete prototype property.
emp1 object doesn't have company as its own property. You can test it like:
However, we can delete company property directly from Employee object using delete Employee.company or we can also delete from emp1 object using __proto__ property delete emp1.__proto__.company .
Q22 : What will the following code output?
This will surprisingly output false because of floating point errors in internally representing certain numbers. 0.1 + 0.2 does not nicely come out to 0.3 but instead the result is actually 0.30000000000000004 because the computer cannot internally represent the correct number. One solution to get around this problem is to round the results when doing arithmetic with decimal numbers.
Q23 : When would you use the bind function?
The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
A good use of the bind function is when you have a particular function that you want to call with a specific this value. You can then use bind to pass a specific object to a function that uses a this reference.
Q24 : Write a recursive function that performs a binary search
Q25 : describe the revealing module pattern design pattern.
A variation of the module pattern is called the Revealing Module Pattern . The purpose is to maintain encapsulation and reveal certain variables and methods returned in an object literal. The direct implementation looks like this:
An obvious disadvantage of it is unable to reference the private methods
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript Coding Questions and Answers
JavaScript is the most commonly used interpreted, and scripted Programming language. It is used to make web pages, mobile applications, web servers, and other platforms. Developed in 1995 by Brendan Eich. Developers should have a solid command over this because many job roles need proficiency in JavaScript .
We will see the Top 50 JavaScript Coding questions and answers including basic and medium JavaScript coding questions and answers. In this article, we will cover everything like JavaScript core concepts- arrays, strings, arrow functions, and classes. These Top 50 coding questions and answers will help you to improve your coding concept in JavaScript.
Basic JavaScript Coding questions and answers
1. write a program to reverse a string in javascript..
This code splits the string into an array of characters using the split() method, reverses the array, and joins it back into a string using the join() method.
2. Write a Program to check whether a string is a palindrome string.
- A palindrome is a word that reads the same word from forward and backward. This ignores spaces and capitalization.
- The code below checks if a string is a palindrome by reversing it and comparing it to the original string. If both are equal, it returns true otherwise, it is false. "GFG" is a palindrome string so it returns true.
3. Find the largest number in an array in JavaScript.
Using a for loop:.
The code defines a function findLargest that finds the largest number in an array. It starts by assuming the first element is the largest, then iterates through the array. If a larger number is found, it updates the largest value. Finally, it returns the largest number which is 100.
Using the spread operator (...) or Math.max:
The findLargest function uses Math.max() to find the largest number in an array. The spread operator (...arr) expands the array elements so Math.max() can evaluate each value. In the given code, it returns 100, the largest number from [99, 5, 3, 100, 1].
4. How Remove the first element from an array in JavaScript?
The code initializes an array arr with values [5, 6, 7]. The slice(1) method creates a new array, excluding the first element (5), resulting in [6, 7]. Finally, console.log(arr) outputs the modified array [6, 7] to the console.
5. Write a Program to use a callback function?
This code defines a greet function that takes two arguments- a name and a callback function. It calls the callback with a greeting message using the name. When greet('Geek', message => console.log(message)) is executed, it prints "Hello, Geek!" as output.
6. Write a code to create an arrow function?
The code defines an arrow function add that takes two arguments a and b and returns their sum (a + b). When console.log(add(6, 2)) is executed, it calls the add function with 6 and 2, and prints the result which is 8, to the console.
7. Write a Program to add a property to an object?
The code creates an object obj with a name property set to 'Riya'. Then, it adds a new property age with the value 21. Finally, console.log(obj) prints the updated object, which now contains both name and age properties: { name: 'Riya', age: 21 }.
8. Write a Program to delete a property from an object?
In this code, an object obj with properties name and age is created. The delete keyword removes the age property from the object. After deletion, console.log(obj) outputs the object, which now only contains the name property: { name: 'Riya' }.
9. What will be the output of the given code?
The code uses the reduce method on the array [1, 2, 3] to sum its elements. It takes two parameters, a (accumulator) and b (current value), adding them together. The final result 6 is printed to the console, representing the total of the array's numbers.
10. What will be the output of the given code?
The code console.log('gfg'.repeat(3)); uses the repeat() method to create a new string by repeating the string 'gfg' three times. The output will be 'gfgfgfg' which is displayed in the console. This method is useful for printing repeated text easily.
11. What will be the output of the given code?
The code console.log(1 + '2'); adds the number 1 and the string '2'. In JavaScript, when a number and a string are combined with +, the number is converted to a string. Finally, it results in the string '12', which is then prints to the console.
12. What will be the output of the given code?
'6' is a string, so when you use the '-' operator with string and number, JavaScript convert the string to a number automatically which is called type coercion. '6' gets converted to the number 6, then 6 - 1 = 5. So, 5 is the answer.
13. What will be the output of the given code?
'1' is a integer and '1' is a string. Strict equality operator(===) check both the type and the value. They look similar but they have different data type. So =, the answer in false.
14. What will be the output of the given code?
'null' represents the absence of any value and 'undefined' represents a variable that has been declared but not assigned any value. The answer is true because JavaScript treats them equal because of '==' loose equality operator.
15. Write a Program to find a sum of an array?
The sumArray function takes an array arr as input and initializes a variable sum to 0. It loops through each element of the array, adding each element's value to sum. Finally, it returns the total sum which is 33.
16. Write a Program to check if a number is prime or not?
The isPrime() function checks if a number num is prime. It returns false for numbers less than or equal to 1. It loops starts from 2 to num - 1, checking if num is divisible by any number in that range. If it is, it returns false otherwise, it returns true.
17. Write a Program to print Fibonacci sequence up to n terms?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1.
The fibonacci function generates the Fibonacci sequence up to n terms. It initializes two variables num1 (0) and num2 (1). In a loop, it prints num1, then calculates the next number as the sum of num1 and num2, updating them for the next iteration. Here, it prints 7 terms.
18. Write a Program to find factorial of a number?
The factorial function calculates the factorial of a given number num. It initializes answer to 1, then multiplies it by each integer from 2 to num in a loop. Finally, it returns the computed factorial. The console.log statement prints the factorial of 7, which is 5040.
19. Calculate the Power of a Number in JavaScript?
The power function takes two arguments- base and exponent. It calculates the result of raising base to the power of exponent using the exponentiation operator **.
20. Write a Program to print the frequency of elements in an array?
The frequency function counts how many times each number appears in an array. It creates an empty object freq, iterates through the array, and either increments the count for existing numbers or adds new numbers with a count of 1. Finally, it returns the freq object with the counts.
Medium JavaScript Coding questions and answers
21. write a program to count the occurrences of a character in a string in javascript, using split() method.
The countChar() function counts how many times a specified character (char) appears in a string (str). It splits the string into an array using the character, then returns the length of the array (length-1), which gives the count of the character. The given code counts 'G' in 'GeeksForGeeks'.
Using a for loop
The countChar() function counts how many times a given character (char) appears in a given string (str). It initializes a counter (count) to zero, iterates through each character in the string, increments the counter when it finds a match and returns the total count.
22. Write a Program to convert Celsius to Fahrenheit in JavaScript?
We are using the formula Fahrenheit=(Celsius×9/5)+32 to convert Celsius to Fahrenheit.
23. Write a Program to convert Fahrenheit to Celsius in JavaScript?
We are using the formula Celsius=(Farhrenheit-32)*5/9 to convert Fahrenheit to Celsius.
24. Write a Program to sort an array in Ascending Order in JavaScript?
The sortArray function sorts an array in ascending order using a nested loop. It compares each element with the others and swaps them if they are out of order. After iterating through the array, it returns the sorted array. For example, [5, 3, 8, 1] becomes [1, 3, 5, 8].
25. write a Program to sort an array in Descending Order in JavaScript?
The code sorts an array in descending order using a bubble sort algorithm. It repeatedly swaps adjacent elements if they are in the wrong order then returning the sorted array.
26. Write a Program to merge two arrays in JavaScript?
The mergeArrays function combines two arrays, arr1 and arr2, by using the concat method, which adds all elements of arr2 to the end of arr1. The function returns the merged array. In the given code [5, 6] and [7, 8] combine to form [5, 6, 7, 8].
27. Find the Intersection of Two Arrays in JavaScript?
In the given code set is used to store a unique values from arr2 then filter checks each element in arr1 to see if it is also exist in the set and keeping only those that matches. The output is an array of common elements from both arr1 and arr2.
28. Find the Union of Two Arrays in JavaScript?
The arrayUnion function merges two arrays (arr1 and arr2) using the spread operator, combines them into a single array, and removes duplicates using Set. It then returns the unique values as a new array. In the given code it outputs [1, 2, 3, 4].
29. Check if a Number is Even or Odd in JavaScript?
The function isEven(num) checks if a number is even by dividing it by 2. If the remainder (num % 2) is 0, the function returns true, means the number is even. Otherwise, it returns false. console.log(isEven(10)) prints true because 10 is even number.
30. Write a Program to find the minimum value in an array in JavaScript?
Using for loop.
The function findMin() takes an array and finds the smallest value. It starts by assuming the first element is the minimum value, then loops through the array, comparing each element. If a smaller value is found, it updates min. value Finally, it returns the smallest value which is -1.
Using Math.min() method
This code defines a function findMin() that takes an array arr as input. It uses Math.min(...arr) to find and return the minimum value from the array by spreading its elements. In this code, it finds -1 as the smallest number in the array [5, 10, -1, 8].
31. Check if a String Contains Another String in JavaScript?
The containsSubstring function checks if a substring exists within a given string. It uses indexOf to search for the substring inside the string. If found, it returns true otherwise, it returns false. In the code, it finds "For" in "GeeksForGeeks."
32. Find the First Non-Repeated Character in a String in JavaScript?
This code finds the first non-repeated character in a string. It first counts how many times each character appears, then checks the string again to return the first character that appears only once. If no non-repeated character is found, it returns null, but in the given string 'F' is a non-repeated character so the output is 'F'.
33. Find the Longest Word in a String in JavaScript?
This code finds the longest word in a given string. It splits the string into an array of words, then iterates through the array. For each word, it checks if its length is greater than the current longest word, if yes then it updating the longest word accordingly. Finally, it returns the longest word.
34. Capitalize the First Letter of Each Word in a Sentence in JavaScript?
The function capitalizeFirstLetter() takes a sentence as input, splits it into an array of words, and capitalizes the first letter of each word. It uses a loop to modify each word and then joins the words back into a sentence, then returning the result.
35. Convert an Array of Strings to Uppercase in JavaScript?
The toUpperCaseArray() function takes an array of strings as input. It creates a new array and converts each string to uppercase using a loop. The uppercase strings are stored in the new array, which is returned. The console logs prints the result for the input ['g', 'f', 'g'].
Hard JavaScript Coding questions and answers
36. write a program to reverse an array in javascript.
The reverseArray function takes an array arr as input and creates a new empty array called reversed[]. It then loops through arr backwards, pushing each element into reversed. Finally it returns the reversed array as output.
37. Get the last element of an array in JavaScript?
The function lastElement() takes an array arr as input and returns its last element. It does this by accessing the element at the index "arr.length - 1", which shows the last position in the array. The console logs prints the last element of the array [6, 2, 9, 5] which is 5.
38. Remove falsy Values from an array in JavaScript?
The falsy values in JavaScript are values which are false, 0, "" (empty string), null, undefined, and NaN.
The removeFalsyValues() function takes an array and filters out falsy values (0, false, ''). It creates an empty array answer[], then iterates through the input array. If an element is truthy, it adds it to answer[]. Then, it returns the array of truthy values
39. Calculate the factorial of a number using recursion in JavaScript?
The factorial function calculates the factorial of a given number n. If n is 0 or 1, it returns 1. Otherwise, it multiplies n by the factorial of n - 1, effectively reducing the problem recursively until it reaches the base case. console.log(factorial(4)) outputs 24.
40. Create an object and print the property?
This code creates an object named "person" with two properties- name ("GFG") and age ( 25). The console.log(person.name) line prints the value of the name property, which is "GFG", as output.
41. Use the map function on an array in JavaScript?
The given creates an array called "numbers" containing the values 5, 6 and 7. It then uses the map function to create a new array "and", where each number is multiplied by 2. Then, it prints the new array, which outputs [10, 12, 14] to the console.
42. Write a Program to create a simple class in JavaScript?
This code defines a class called "Animals" with a constructor that initializes the animal's name. The speak method prints a message including the animal's name and a generic noise. A new instance of Animals, named dog, is created, and its speak method is called, so the output is "Dog makes a noise."
43. Use JSON to parse and stringify data in JavaScript?
The code defines a JSON string jsonData containing a name. It uses JSON.parse() to convert the JSON string into a JavaScript object, storing it in parsedData. Finally, it logs the value of the name property from the object, which outputs "Geeks" to the console.
44. Convert a string to an array of words in JavaScript?
This code defines a string sentence containing "Geeks For Geeks". The split() method divides the string into individual words using spaces as separators then creating an array called "wordsArray" . The resulting array, which contains ["Geeks", "For", "Geeks"], is an output.
45. Write a switch statement code in JavaScript?
This code defines a variable course with the value "javascript". The switch statement checks the value of course. If it matches "javascript", it prints "This is a javascript course" to the console. If it does not match, the default case prints "Not a javascript course" .
46. Check if Two Strings are Anagrams or not in JavaScript?
The areAnagrams() function checks if two strings are anagrams. First it compares their lengths if they are not same then it returns false. Then, it counts character frequencies for each string. Then, it compares these counts. If they match, the strings are anagrams(true), otherwisethey are not anagrams(false) .
47. Find the maximum difference between two numbers in an array in JavaScript?
The maxDifference() function finds the maximum difference between elements in an array, where the larger element comes after the smaller one. It initializes min variable with the first element of an array, then iterates through the array, updating the maxDiff and the minimum value.
48. Remove Duplicates from an Array in JavaScript?
The removeDuplicates function takes an array arr as input and creates a new array "uniqueArray" . It iterates through arr and checking if each element is already in uniqueArray or not. If not, it adds the element in uniqueArray. Finally, it returns uniqueArray which contains only unique values from the original array.
49. Count Vowels in a String in JavaScript?
The countVowels function counts the number of vowels in a given string. It initializes a count variable as 0 and iterates through each character of the string. If a character is found in the vowels string (which includes both lowercase and uppercase vowels), it increments the count variable. Atlast, it returns the total count.
50. Get Unique Characters from a String in JavaScript?
The uniqueCharacters function takes a string as input and finds unique characters. It initializes an empty array, uniqueChars, and iterates through the string. If a character is not already in uniqueChars, it adds it. Finally, it joins the unique characters into a string and returns the string.
Similar Reads
- JavaScript Interview Questions and Answers JavaScript (JS) is the most popular lightweight, scripting, and interpreted programming language. It was developed by Brendan Eich in 1995. JavaScript is well-known as a scripting language for web pages, mobile apps, web servers, and many other platforms. It is essential for both front-end and back- 15+ min read
- JavaScript String Interview Questions and Answers JavaScript (JS) is the world's most popular lightweight programming language, created by Brendan Eich in 1995. It is a must-have skill for web development, mobile apps, web servers, and more. Whether you aim to be a front-end or back-end developer, mastering JavaScript is crucial for landing top job 14 min read
- JavaScript Array Interview Questions and Answers JavaScript Array Interview Questions and Answers contains the list of top 50 array based questions that are frequently asked in interviews. The questions list are divided based on difficulty levels (Basic, Intermediate, and Advanced). This guide covers fundamental concepts, common problems, and prac 15+ min read
- JavaScript Interview Questions and Answers (2024) - Intermediate Level In this article, you will learn JavaScript interview questions and answers intermediate level that are most frequently asked in interviews. Before proceeding to learn JavaScript interview questions and answers – intermediate level, first we learn the complete JavaScript Tutorial, and JavaScript Inte 6 min read
- JavaScript Output Based Interview Questions JavaScript (JS) is the most widely used lightweight scripting and compiled programming language with first-class functions. It is well-known as a scripting language for web pages, mobile apps, web servers, and many more. In this article, we will cover the most asked output-based interview questions 15+ min read
- JavaScript Exercises, Practice Questions and Solutions JavaScript Exercise covers interactive quizzes, track progress, and enhance coding skills with our engaging portal. Ideal for beginners and experienced developers, Level up your JavaScript proficiency at your own pace. Start coding now! A step-by-step JavaScript practice guide for beginner to advanc 4 min read
- Node Exercises, Practice Questions and Solutions Node Exercise: Explore interactive quizzes, track progress, and enhance coding skills with our engaging portal. Ideal for beginners and experienced developers, Level up your Node proficiency at your own pace. Start coding now! #content-iframe { width: 100%; height: 500px;} @media (max-width: 768px) 4 min read
- Difference between Java and JavaScript Java is a statically typed, object-oriented programming language for building platform-independent applications. JavaScript is a dynamically typed scripting language primarily used for interactive web development. Despite similar names, they serve different purposes and have distinct syntax, runtime 5 min read
- 7 Interesting Game Projects To Enhance Your JavaScript Skills JavaScript is indeed one of the most popular, demanded, and used programming languages in the tech world. The language provides significant support for both - client-side and server-side development. Further on, it has a vast range of applications like web development, mobile apps development, game 7 min read
- JavaScript Array Exercise In JavaScript, an Array is a versatile data structure that allows you to store multiple values in a single variable. Arrays can hold different data types, including strings, numbers, objects, and even other arrays. JavaScript arrays are dynamic, which means they can grow or shrink in size. [GFGTABS] 1 min read
- Java Unnamed Classes and Instance Main Methods Java has introduced a significant language enhancement in Java Enhancement Proposal (JEP) 445, titled "Unnamed Classes and Instance Main Methods". This proposal aims to address the needs of beginners, making Java more accessible and less intimidating. Let's delve into the specifics of this proposal 7 min read
- 10 Best JavaScript Project Ideas For Beginners in 2024 In a world where everyone wants to become a developer, let's also talk about the skills required for development. When we talk about development, JavaScript comes on top of the list for programming languages. With so much demand for JavaScript that is used by many developers (65% of the total develo 9 min read
- JavaScript Best Practices for Code Review The Code reviews are an essential part of the software development process helping the teams maintain code quality catch bugs early and ensure adherence to the coding standards. In JavaScript development, code reviews play a crucial role in identifying potential issues improving readability, and pro 3 min read
- JavaScript Online Compiler The JavaScript (JS) Online Compiler and Editor is a free online tool providing an integrated development environment (IDE) for creating and executing JavaScript code. Whether you are a beginner looking to learn JavaScript or an experienced developer want to test snippets of code quickly, an online c 5 min read
- HTML Exercises, Practice Questions and Solutions Are you eager to learn HTML or looking to brush up on your skills? Dive into our HTML Exercises, designed to cater to both beginners and experienced developers. With our interactive portal, you can engage in hands-on coding challenges, track your progress, and elevate your web development expertise. 3 min read
- JavaScript Programming Examples JavaScript is a popular compiled programming language in the world of web development, providing to both new and experienced developers. Now, practicing programming examples is useful for improving your logical and coding skills. JavaScript programming examples, encompassing fundamental syntax like 15 min read
- JavaScript | A medicine to every problem In the world full of different languages it is quite confusing knowing that what to choose and what not to. Most of the times students want to start something but when they search they have a lot of options and a lot of technologies in the market. It is good to have options but sometimes a lot of op 4 min read
- JavaScript Number Programming Examples Javascript Numbers are primitive data types. Unlike other programming languages, Both integers and floating-point values are represented by JavaScript's number type. The IEEE-754 format is the one that is technically used by the JavaScript number type. Here, you don’t need int, float, etc, to declar 3 min read
- JavaScript Project Ideas with Source Code JavaScript is a lightweight, cross-platform programming language that powers dynamic and interactive web content. From real-time updates to interactive maps and animations, JavaScript brings web pages to life. This article presents 80+ JavaScript projects with source code and ideas, suitable for all 3 min read
- Web Technologies
- Web-Tech Blogs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Precision in Payroll - Acing Taxes and Compliance
Webinar HR Metrics Magic: Transforming Insights into Actions Register Now
- Interview Questions
Top 50 JavaScript coding Interview Questions and Answers
Table of Contents
JavaScript is one of the widely used programming languages and used to create engaging and dynamic websites. Due to its vast scope, JavaScript interviews can be challenging . However, thorough preparation can help overcome these challenges. An interviewee should familiarize themselves with the most basic concepts to the most complex libraries and frameworks.
These top 50 JavaScript interview questions and answers will help an intervie wee to thoroughly practice and prepare for their interview.
Top 50 JavaScript Coding Interview Questions
Basic javascript coding questions.
Basic JavaScript questions cover concepts like data types, variables and scoping, array, string manipulation, OOP (Object Oriented Programming), control flow, error handling, DOM manipulation, and asynchronous programming. The basic JavaScript coding interview questions are:
1. Write a JavaScript function to calculate the sum of two numbers.
When managers ask this question, they are looking for the candidate’s basic understanding of JavaScript. They assess their understanding of basic syntax along with problem-solving skills. This also helps evaluate the candidate’s coding style and attention to detail.
I would take two parameters and the following function can be used to calculate the sum of any 2 numbers that are passed as arguments. function sumOfTwoNumbers(a, b) { return a + b; }
2. Write a JavaScript program to find the maximum number in an array.
A hiring manager asks this question to analyze the candidate’s ability to write clear and efficient code. It’s crucial for candidates to explain the code step-by-step while demonstrating bug-free code.
function findMaxNumber(arr) { return Math.max(…arr); }
3. Write a JavaScript function to check if a given string is a palindrome (reads the same forwards and backwards).
The interviewer is looking for the candidate’s familiarity with loop constructs, JavaScript string methods, and other basic JavaScript syntax. They will evaluate the candidate’s skills based on the approach used to solve the palindrome problem.
function isPalindrome(str) { return str === str.split(”).reverse().join(”); }
4. Write a JavaScript program to reverse a given string.
Hiring managers are expecting an accurate solution that demonstrates the interviewee’s proficiency in JavaScript programming.
const reverseString = (str) => str.split(”).reverse().join(”);
5. Write a JavaScript function that takes an array of numbers and returns a new array with only the even numbers.
Interviewers are looking for candidates who can not only clearly explain the solution along with the code, but also show the ability to think logically and articulate their thought processes.
By using the filter method on the array, I can check if each element is even or not by using the modulus operator (%) with 2. The element is even if the result is 0. This can be included in the new array. function filterEvenNumbers(numbers) { return numbers.filter(num => num % 2 === 0); }
6. Write a JavaScript program to calculate the factorial of a given number.
By asking this question, managers aim to assess the candidate’s algorithmic thinking and understanding of JavaScript programming. The interviewer expects the candidate to demonstrate their knowledge of the factorial concept.
A factorial number is the product of all positive integers, which are equal to or less than the given number. function factorial(number) { if (number === 0 || number === 1) { return 1; } else { return number * factorial(number – 1); } }
7. Write a JavaScript function to check if a given number is prime.
Interviewers can analyze the candidate’s knowledge of JavaScript algorithms and mathematical concepts. They expect the candidate to translate a mathematical concept into functional code.
To check if a given number is prime, loop from 2 to the square root of the number. If any integer evenly divides it, the number is not prime. function isPrime(num) { if (num <= 1) return false; for (let i = 2; i <= Math.sqrt(num); i++) { if (num % i === 0) return false; } return true; }
8. Write a JavaScript program to find the largest element in a nested array.
When asking this question, interviewers are looking for the candidate’s ability to handle nested data structures and apply their knowledge of conditional statements, arrays, and loops. Candidates must apply their knowledge to real-world scenarios.
function findLargestElement(nestedArray) { let largest = nestedArray[0][0]; for (let arr of nestedArray) { for (let num of arr) { if (num > largest) { largest = num; } } } return largest; }
9. Write a JavaScript function that returns the Fibonacci sequence up to a given number of terms.
This question helps hiring managers assess the interviewee’s understanding of fundamental algorithms in JavaScript. They expect the candidate to consider edge cases and handle errors.
function fibonacciSequence(numTerms) { if (numTerms <= 0) return []; if (numTerms === 1) return [0]; let sequence = [0, 1]; while (sequence.length < numTerms) { let nextNumber = sequence[sequence.length – 1] + sequence[sequence.length – 2]; sequence.push(nextNumber); } return sequence; }
10. Write a JavaScript program to convert a string to title case (capitalize the first letter of each word).
Interviewers analyze the candidate’s ability to break down a problem into manageable steps and demonstrate knowledge of string manipulation, looping, and basic JavaScript functions.
function toTitleCase(str) { return str.replace(/\b\w/g, l => l.toUpperCase()); }
A dvanced JavaScript coding interview questions
Advanced JavaScript coding includes various complex concepts and techniques. Such key concepts are often tested in JavaScript interviews. Some of the concepts are – closure and scope, prototypal inheritance, functional programming , design patterns, memory management, ES6+ features, and many more.
1. Implement a debounce function in JavaScript that limits the frequency of a function’s execution when it’s called repeatedly within a specified time frame.
Interviewers expect the candidate to showcase their ability to clearly explain the purpose of the debounce function and its usage in scenarios where function calls need to be controlled. They are looking for the person’s ability to articulate technical concepts clearly.
By delaying the execution of the debounce function until the specified time frame has passed, the frequency can be limited. function debounce(func, delay) { let timer; return function() { clearTimeout(timer); timer = setTimeout(func, delay); }; }
2. Write a function that takes an array of objects and a key, and returns a new array sorted based on the values of that key in ascending order.
By asking this question, hiring managers analyze how well the candidate can discuss the sorting algorithm and its time complexity. It’s also crucial for candidates to demonstrate their code’s robustness.
The following function takes an array of objects and a key to sort the array based on the values in ascending order. function sortByKey(arr, key) { return arr.sort((a, b) => a[key] – b[key]); }
3. Implement a deep clone function in JavaScript that creates a copy of a nested object or array without any reference to the original.
Hiring managers want to assess the interviewee’s skill to handle complex coding tasks and understand the concept of avoiding reference issues while cloning.
By using two methods together and creating a deep clone, I can serialize the object to a JSON string. I would then parse it back into a new object, thereby removing any reference to the original object. function deepClone(obj) { return JSON.parse(JSON.stringify(obj)); }
4. Write a recursive function to calculate the factorial of a given number.
Interviewers expect the candidate to write a concise recursive function that handles edge cases. Candidates must show their understanding of how recursion works to avoid infinite loops or stack overflow errors.
function factorial(num) { if (num <= 1) return 1; return num * factorial(num – 1); }
5. Implement a function that takes two sorted arrays and merges them into a single sorted array without using any built-in sorting functions.
When interviewers ask this question, they seek to assess the knowledge of algorithms and efficiency in handling sorted data. They also look for the ability to think of and execute a correct solution.
I can implement a function that can efficiently merge two sorted arrays. function mergeSortedArrays(arr1, arr2) { return […arr1, …arr2].sort((a, b) => a – b); }
6. Write a function that checks if a given string is a palindrome, considering only alphanumeric characters and ignoring case.
Interviewers analyze the interviewee’s approach to execute code and demonstrate familiarity with handling case-sensitive and alphanumeric checks, regular expressions, and JavaScript string methods.
function isPalindrome(str) { const cleanStr = str.replace(/[^a-zA-Z0-9]/g, ”).toLowerCase(); const reversedStr = cleanStr.split(”).reverse().join(”); return cleanStr === reversedStr; }
7. Create a JavaScript class for a linked list with methods to insert a node at the beginning, end, or at a specific position, and to delete a node from a given position.
By asking this question, interviewers can evaluate how well a candidate can design and implement a class for a linked list while also presenting their problem-solving skills .
I would implement a linked list with methods to insert a node at the beginning, end, and at specific positions. Then, I would delete a node from a given position.
8. Implement a function that flattens a nested array in JavaScript, converting it into a single-level array.
Managers can gauge the candidate’s logical thinking skills and capability to handle complex data structures. Interviewees should demonstrate their knowledge of loops, recursion, and arrays.
const flattenArray = (nestedArray) => { return nestedArray.flat(Infinity); };
9. Write a function that determines if two strings are anagrams of each other
When interviewers present this question, they aim to measure how well the candidate can use appropriate string-related methods and identify anagrams accurately.
function areAnagrams(str1, str2) { return str1.split(“”).sort().join(“”) === str2.split(“”).sort().join(“”); }
10. Create a JavaScript function that returns the Fibonacci sequence up to a given number, utilizing memoization for optimized performance.
Interviewees are expected to show their proficiency in OOP and familiarity with recursion and memoization. They can also determine the candidate’s attention to detail in class design and organizing code.
By creating a function that uses an array to store the computed values, a Fibonacci sequence can be generated. function fibonacciWithMemoization(n) { let memo = [0, 1]; for (let i = 2; i <= n; i++) { memo[i] = memo[i – 1] + memo[i – 2]; } return memo; }
Common JavaScript coding interview questions
Some of the common JavaScript coding interview questions typically cover these topics: checking for palindrome, finding missing/largest numbers, object manipulation, removing duplicates, merging, etc.
1. Write a function to check if a given string is a palindrome.
Hiring managers review how well a candidate can handle edge cases while handling case sensitivity, punctuation, and whitespace.
This function takes a string as input to convert it into lowercase and then compares it with its reverse. The string can be deemed a palindrome if the two match. function isPalindrome(str) { return str.toLowerCase() === str.toLowerCase().split(”).reverse().join(”); }
2. Implement a function to reverse a string without using the built-in reverse() method.
Hiring managers aim to analyze the candidate’s knowledge of string manipulation in JavaScript while also measuring their ability to think of alternative solutions.
I would use a for lopp to iterate through the characters from the end to the beginning. By appending the character to a new string, it results in the reversed output. function reverseString(str) { let reversed = ”; for (let i = str.length – 1; i >= 0; i–) { reversed += str[i]; } return reversed; }
3. Given an array of numbers, write a function to find the largest and smallest numbers in the array.
By presenting the candidates with this question, managers can gauge how well a candidate is familiar with basic JavaScript functions and array manipulation.
I would use the following functions to find the smallest and largest numbers in the array. function findMinMax(arr) { let min = Math.min(…arr); let max = Math.max(…arr); return [min, max]; }
4. Write a function that takes an array of integers as input and returns a new array with only the unique elements.
Hiring managers can evaluate the candidate’s knowledge of JavaScript functions, array handling capabilities, and communicating technical concepts.
function getUniqueElements(arr) { return Array.from(new Set(arr)); }
5. Implement a function to find the factorial of a given number.
Interviewers can determine the candidate’s capability to execute functional code and ability to handle input validation and edge cases. Interviewers also assess the ability to use concise and effective code and provide efficient code implementation.
function factorial(number) { if (number === 0 || number === 1) return 1; return number * factorial(number – 1); }
6. Write a function that determines if a given number is prime or not.
By asking this question, interviewers can understand how good the candidate is proficient in math operations and JavaScript logic. The interviewee should excute a clean and optimized solution that is efficient.
function isPrime(num) { if (num <= 1) return false; for (let i = 2; i <= Math.sqrt(num); i++) { if (num % i === 0) return false; } return true; }
7. Implement a function to find the sum of all the numbers in an array.
Such a question helps understand if the interviewee can manipulate arrays and handle numeric values. This also helps managers assess problem-solving capabilities and ability to pay attention to code efficiency.
I would use the reduce method to implement the following function: function findSum(arr) { return arr.reduce((sum, num) => sum + num, 0); }
8. Given a string, write a function to count the occurrences of each character in the string.
Hiring managers expect the candidate to be familiar with string manipulation and loop constructs. When they ask this question, they can evaluate whether the candidate knows data structures.
function countCharacterOccurrences(str) { const charCount = {}; for (let char of str) { charCount[char] = (charCount[char] || 0) + 1; } return charCount; }
9. Implement a function to remove duplicates from an array.
When interviewers present the candidate with this question, they can gauge the level of understanding a candidate has regarding array methods and different approaches to solve the problem.
The following function duplicates from an array by converting it into a Set. This automatically removes duplicates. Next, the function converts the Set back into an array. function removeDuplicates(arr) { return Array.from(new Set(arr)); }
10. Write a function that sorts an array of numbers in ascending order.
Interviewees must show their knowledge of bubble sort, merge sort, sorting algorithms, and other approaches. The HR manager aims to measure the capability to execute strong algorithms and handle edge cases.
I can solve this by using JavaScript’s built-in sort method. function ascendingSort(numbers) { return numbers.sort((a, b) => a – b); }
Tricky JavaScript coding questions
By asking tricky JavaScript coding questions, managers can assess problem—solving skills, JavaScript concepts, and critical thinking . These go beyond syntax knowledge and require the candidate to think creatively and logically to solve problems.
1. Write a function that reverses the order of words in a sentence without using the built-in reverse() method.
This question not only assesses the creativity of the candidates but also helps hiring managers understand how well a candidate can come up with a clean and understandable solution.
function reverseSentence(sentence) { const words = sentence.split(‘ ‘); const reversedWords = words.reverse(); return reversedWords.join(‘ ‘); }
2. Implement a function that checks if a given string is a palindrome (reads the same forwards and backwards) while ignoring whitespace and punctuation.
Interviewers can gauge the interviewee’s capability to handle whitespace and punctuation gracefully while also maintaining the palindrome-checking logic. Candidates must express their knowledge of regular expressions or any other efficient approach.
function isPalindrome(str) { const cleanedStr = str.replace(/[^\w]/g, ”).toLowerCase(); const reversedStr = cleanedStr.split(”).reverse().join(”); return cleanedStr === reversedStr; }
3. Write a function that takes an array of integers and returns the largest difference between any two numbers in the array.
Candidates should demonstrate their approach to finding the maximum difference between the array elements to handle edge cases and invalid inputs.
function largestDifference(arr) { let min = arr[0]; let maxDiff = 0; for (let i = 1; i < arr.length; i++) { if (arr[i] < min) { min = arr[i]; } else { const diff = arr[i] – min; if (diff > maxDiff) { maxDiff = diff; } } } return maxDiff; }
4. Implement a function that removes duplicates from an array, keeping only the unique elements.
Interviewers can analyze how well a candidate can effectively communicate code explanations and their familiarity with algorithmic efficiency.
function removeDuplicates(arr) { return arr.filter((item, index) => arr.indexOf(item) === index); }
5. Write a function that accepts a number and returns its factorial (e.g., factorial of 5 is 5 x 4 x 3 x 2 x 1).
By presenting this question in the interview, hiring managers can assess the capability of the candidate to handle numeric calculations. They can also determine how well the interviewee can pay attention to handling edge cases, if applicable.
function factorial(num) { if (num === 0 || num === 1) { return 1; } else { return num * factorial(num – 1); } }
6. Implement a function that flattens a nested array into a single-dimensional array.
Interviewers expect the candidates to demonstrate their ability to work with complex data structures and use appropriate techniques to accomplish tasks.
function flattenArray(arr) { return arr.flat(); }
7. Write a function that checks if a given string is an anagram of another string (contains the same characters in a different order).
Candidates should showcase how well they can handle complex algorithms and logic. Interviewers are specifically looking for knowledge in string methods, data structures, and loop constructs.
function isAnagram(str1, str2) { const sortedStr1 = str1.split(”).sort().join(”); const sortedStr2 = str2.split(”).sort().join(”); return sortedStr1 === sortedStr2; }
8. Implement a function that finds the second smallest element in an array of integers.
Interviewers can measure the candidate’s problem-solving skills and their understanding of conditional statements, loops, and arrays.
function secondSmallest(arr) { const sortedArr = arr.sort((a, b) => a – b); return sortedArr[1]; }
9. Write a function that generates a random alphanumeric string of a given length.
By asking this question, interviewers can understand how well a candidate can ensure the function produces a reliable and consistent random output.
function generateRandomString(length) { const characters = ‘ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789’; let result = ”; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * characters.length); result += characters.charAt(randomIndex); } return result; }
10. Implement a function that converts a number to its Roman numeral representation.
Hiring managers can gauge a candidate’s capability to implement coding solutions and create an efficient algorithm.
function toRomanNumeral(number) { // Implement your code here }
JavaScript array coding questions
JavaScript array coding interview questions are technical questions asked to gauge candidates’ ability to work with arrays along with their familiarity with fundamental data structures.
1. Write a function that returns the sum of all numbers in an array.
By asking such a question, hiring managers can evaluate whether the candidate would be able to perform common tasks and solve basic coding challenges.
function sumArray(arr) { return arr.reduce((total, num) => total + num, 0); }
2. Implement a function that finds the maximum number in an array.
Depending on the candidate’s answer, the manager can determine how effectively the interviewee can work with arrays. The managers can also understand the capability to communicate technical solutions.
function findMaxNumber(arr) { let max = arr[0]; for (let i = 1; i < arr.length; i++) { if (arr[i] > max) { max = arr[i]; } } return max; }
3. Write a function that returns a new array containing only the unique elements from an input array.
The hiring manager is specifically looking for candidates who can demonstrate an understanding in data manipulation and JavaScript arrays. Additionally, interviewers evaluate how well the candidate strives for an optimized solution without duplicate elements.
function getUniqueElements(inputArray) { return […new Set(inputArray)]; }
4. Implement a function that returns the average value of numbers in an array.
By asking this question, hiring managers can assess the candidate’s knowledge of arithmetic operations, array manipulation, and looping.
function calculateAverage(numbers) { let sum = 0; for (let number of numbers) { sum += number; } return sum / numbers.length; }
5. Write a function that sorts an array of strings in alphabetical order.
When interviewers present this question in the interview, they expect the candidate to be familiar with sorting algorithms and JavaScript array manipulation.
function sortStrings(arr) { return arr.slice().sort(); }
6. Implement a function that finds the index of a specific element in an array. If the element is not found, the function should return -1.
Interviewers aim to gauge the candidate’s proficiency in use of array methods, handling edge cases, and in JavaScript syntax. Candidates must implement the function proper error handling.
function findElementIndex(arr, element) { const index = arr.indexOf(element); return index !== -1 ? index : -1; }
7. Write a function that removes all falsy values (false, null, 0, “”, undefined, and NaN) from an array.
Candidates must showcase communication skills and explain their solutions logically. Interviewers analyze the interviewee’s ability to write a function that filters false values from an array.
function removeFalsyValues(arr) { return arr.filter(Boolean); }
8. Implement a function that merges two arrays into a single array, alternating elements from each array.
Hiring managers determine a candidate’s ability to craft efficient algorithms and knowledge of array manipulation.
function mergeArrays(array1, array2) { const mergedArray = []; const maxLength = Math.max(array1.length, array2.length); for (let i = 0; i < maxLength; i++) { if (i < array1.length) mergedArray.push(array1[i]); if (i < array2.length) mergedArray.push(array2[i]); } return mergedArray; }
9. Write a function that finds the second largest number in an array.
Such a question reveals to the interviewers how well the candidate can use loops and array methods, work with them, and utilize logic to find solutions.
function findSecondLargest(arr) { arr.sort((a, b) => b – a); return arr[1]; }
10. Implement a function that groups elements in an array based on a given condition. For example, grouping even and odd numbers into separate arrays.
When interviews ask this question, they aim to evaluate a candidate’s understanding of concepts like array methods, conditional statements, and other technical concepts. Candidate should demonstrate good coding style.
function groupByCondition(arr, condition) { return [ arr.filter(element => condition(element)), arr.filter(element => !condition(element)) ]; }

Tips to prepare for a JavaScript coding interview
There are 5 tips a candidate should keep in mind when attending a JavaScript coding interview:
- Master JavaScript basics: Candidates must have a solid understanding of core JavaScript fundamentals, including variables, loops, conditionals, objects, and data types. Additionally, practicing coding skills is important.
- Explore popular libraries and frameworks: Familiarizing oneself with the most common JavaScript libraries and frameworks like Vue.js, React, etc., helps understand key concepts.
- Practice whiteboard Java coding: Java coding skills can further be sharpened by practicing whiteboard coding. Candidates should solve coding challenges and explain their thought processes loudly. They should mainly focus on simplicity, clarity, and efficiency.
- Test code : After writing Java solutions, test it with various inputs and ensure it works correctly and can handle edge cases. Consider the time complexity of the solutions and aim for efficiency.
- Review JavaScript projects : Discussing JavaScript projects in the interview is essential. Interviewees should explain their approach clearly and how they overcame the challenges.
There are some red flags hiring managers should watch out for in a JavaScript coding interview:
- Lack of basic JavaScript knowledge
- Providing inefficient solutions
- No practical problem-solving abilities
- Limited knowledge of asynchronous programming
- Copy-pasting code
- Inability to articulate thought processes or provide a clear explanation of their approach to the problem
- Unfamiliarity with Modern JavaScript
- Failure to provide error handling and consider edge cases
Candidates are evaluated majorly on their understanding of core JavaScript concepts and skills. By mastering the fundamentals and practicing, candidates can crack their interview. Also, it’s important to focus on being clear, accurate , and honest in the interview.
Related Interview Questions
- Java programming interview questions and Answers
- Top 40 Java Interview Questions and Answers 2023
Related Job Descriptions
- Java Developer Job Description
- Full Stack Developer Job Description Template
Subscribe to our Newsletter
Curated HR content delivered to you, bi-monthly!

We use cookies to ensure you get the best experience. Check our " privacy policy ”
50 Top JavaScript Interview Questions With Example Answers
Review these common JavaScript interview questions and answers and practice your coding fundamentals with this guide to ace your next interview.

Preparing for a JavaScript interview requires a lot of work. It’s important to be well-versed in the fundamentals but you also should have some grasp on how to debug JavaScript code , what some of the advanced functions are and how to build projects in it.
Common JavaScript Interview Questions
- What are the different data types in JavaScript?
- What is hoisting in JavaScript?
- What is the difference between null and undefined?
- What are closures in JavaScript?
- What is a callback function in JavaScript?
- What are promises in JavaScript?
- What is the purpose of the setTimeout() function in Javascript?
- How can you check if an array includes a certain value?
- How can you remove duplicates in an array?
- What is the purpose of async and await in JavaScript?
Below are some tips for preparing for the interview along with some common questions and answers to help you ace your next interview.
JavaScript Interview Questions and Answers With Examples
JavaScript interview questions range from the basics like explaining the different data types in JavaScript to more complicated concepts like generator functions and async and await. Each question will have answers and examples you can use to prepare for your own interview.
More on JavaScript How to Use the Ternary Operator in JavaScript
JavaScript Fundamentals
1. what is javascript.
JavaScript is a high-level, interpreted programming language that makes it possible to create interactive web pages and online apps with dynamic functionality. Commonly referred to as the universal language, Javascript is primarily used by developers for front-end and back-end work.
2. What Are the Different Data Types in JavaScript?
JavaScript has six primitive data types :
- Number
It also has two compound data types:
3. What Is Hoisting in JavaScript?
Hoisting is a JavaScript concept that refers to the process of moving declarations to the top of their scope. This means that variables and functions can be used before they are declared, as long as they are declared before they are used in a function.
For example, the following code will print "Hello, world!" even though the greeting variable is not declared until after the console.log() statement.
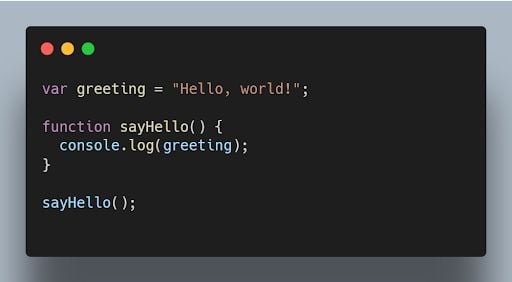
4. What Is the Difference Between null and undefined?
null is an assignment value that represents no value or an empty value , while undefined is a variable that has been declared but not assigned a value.
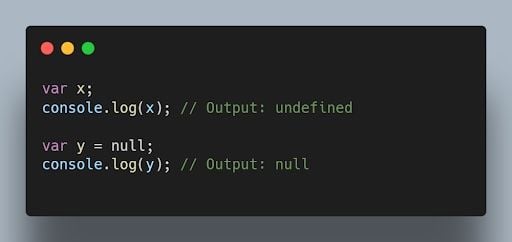
5. Why Do We Use the Word “debugger” in JavaScript?
The word “debugger” is used in JavaScript to refer to a tool that can be used to step through JavaScript code line by line. This can be helpful for debugging JavaScript code, which is the process of finding and fixing errors in JavaScript code. To use the debugger , you need to open the JavaScript console in your browser. Then, you can use debugger commands to comb through your code line by line.
It's essential to know debugging techniques as well as the more general ideas behind code optimization and speed improvement. In addition to operating smoothly, efficient code significantly enhances the user experience.
For example, the following code will print the value of the x variable at each step of the debugger .
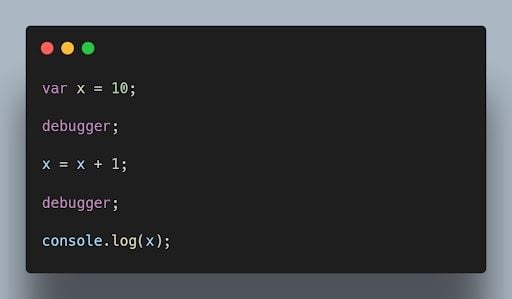
6. What Is the Purpose of the “this” Keyword in JavaScript?
The this keyword refers to the object that is executing the current function or method. It allows access to object properties and methods within the context of that object.
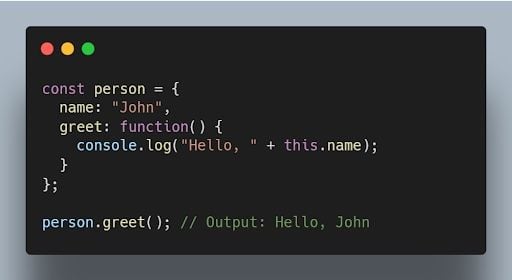
7. What Is the Difference Between == and === Operators in JavaScript?
The equality == operator is a comparison operator that compares two values and returns true if they are equal. The strict equality === operator is also a comparison operator, but it compares two values and returns true only if they are equal and of the same type.
For example, the following code will return true, because the values of the x and y variables are equal.
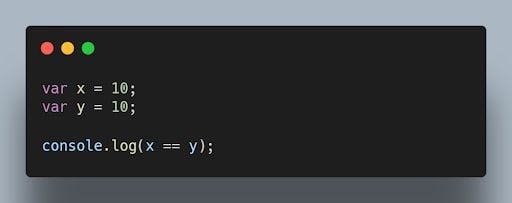
8. What Is the Difference Between “var” and “let” Keywords in JavaScript?
The var and let keywords are both used to declare variables in JavaScript. However, there are some key differences between the two keywords.
The var keyword declares a global variable, which means that the variable can be accessed from anywhere in the code. The let keyword declares a local variable, which means that the variable can only be accessed within the block of code where it is declared.
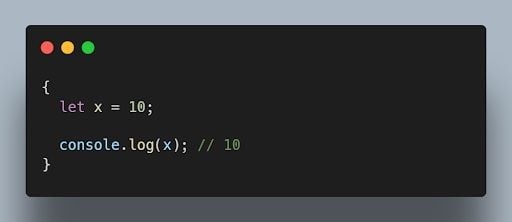
9. What Are Closures in JavaScript?
Closures ( closureFn ) are functions that have access to variables from an outer function even after the outer function has finished executing. They “remember” the environment in which they were created.
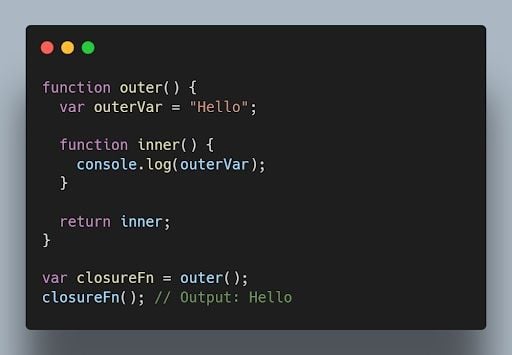
10. What Is Event Delegation in JavaScript?
Event delegation is a technique where you attach a single event listener to a parent element, and that event listener handles events occurring on its child elements. It helps optimize performance and reduce memory consumption.
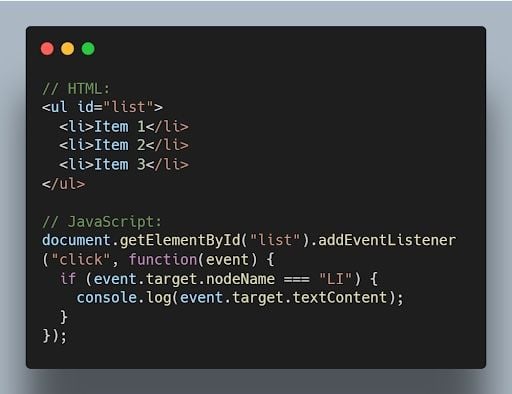
11. What Is the Difference Between “let”, “const”, and “var”?
let and const were introduced in ES6 and have block scope. let is reassignable, and const is non-reassignable. var is function-scoped and can be redeclared and reassigned throughout the function.
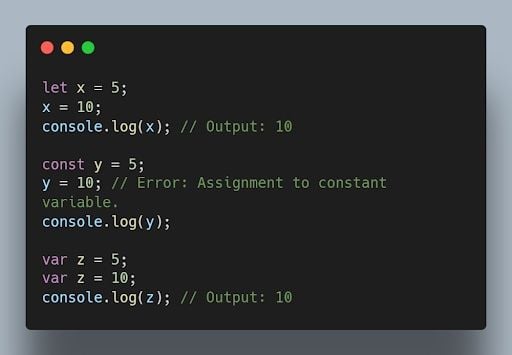
12. What Is Implicit Type Coercion in JavaScript?
Implicit type coercion is a JavaScript concept that refers to the automatic conversion of a value from one type to another. In JavaScript, this conversion follows a priority order that typically begins with strings, then numbers, and finally booleans. If you try to add a string to a number , JavaScript will implicitly coerce the number to a string before performing the addition operation because strings have the highest priority in type coercion.
For example, when you combine the number 5 with the string '10' using the addition operator, the result is the string '510' . This occurs because JavaScript will implicitly convert the number 5 to a string following the priority of coercion, and then concatenate it to the string '10' .
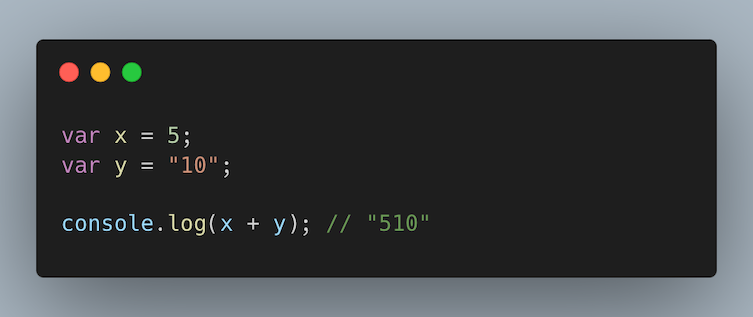
13. Explain the Concept of Prototypes in JavaScript.
Prototypes are a mechanism used by JavaScript objects for inheritance. Every JavaScript object has a prototype, which provides properties and methods that can be accessed by that object.
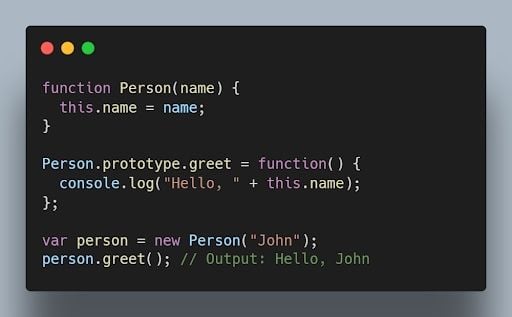
14. What Is the Output of the Following Code?
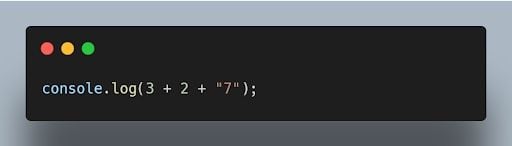
The output will be "57" . The addition operation is performed from left to right, and when a string is encountered, it performs concatenation.
15. How Can You Clone an Object in JavaScript?
There are multiple ways to clone an object in JavaScript. One common method is using the Object.assign() method or the spread operator (...) .
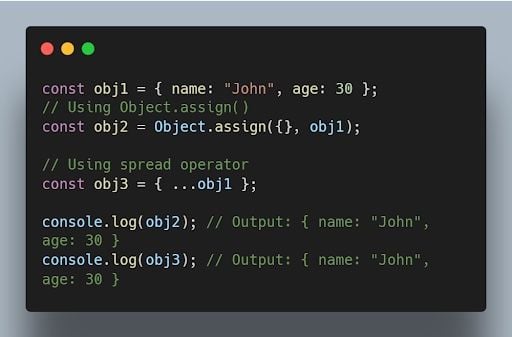
More on JavaScript JavaScript Question Mark (?) Operator Explained
Intermediate JavaScript Concepts
16. what are higher-order functions in javascript.
Higher-order functions are functions that can accept other functions as arguments or return functions as their results. They enable powerful functional programming patterns in JavaScript .
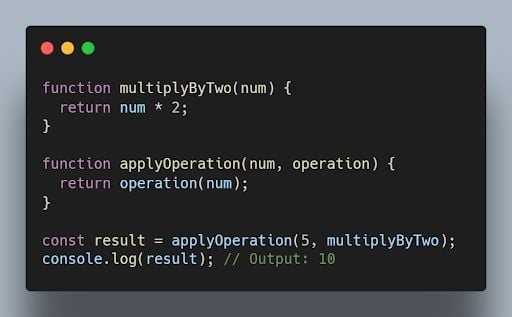
17. What Is the Purpose of the bind() Method in JavaScript?
The bind() method is used to create a new function with a specified this value and an initial set of arguments. It allows you to set the context of a function permanently.
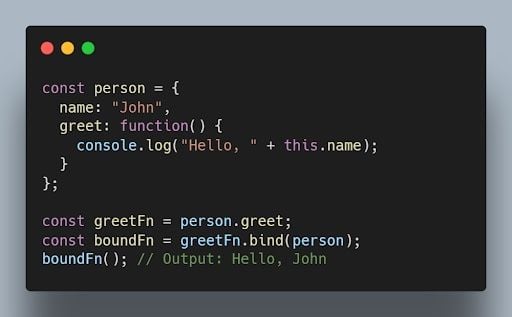
18. What Is the Difference Between Function Declarations and Function Expressions?
Function declarations are defined using the function keyword, while function expressions are defined by assigning a function to a variable. Function declarations are hoisted, while function expressions are not.
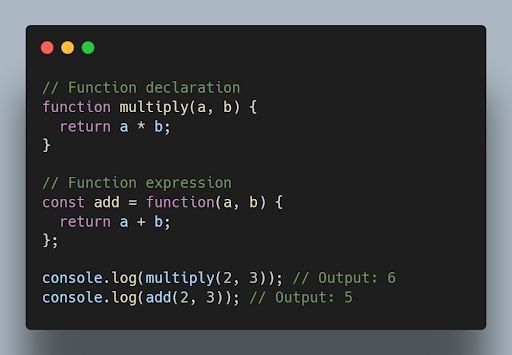
19. What Are the Different Types of Errors in JavaScript?
JavaScript can throw a variety of errors, including:
- Syntax errors: These errors occur when the JavaScript code is not syntactically correct.
- Runtime errors: These errors occur when the JavaScript code is executed and there is a problem.
- Logical errors: These errors occur when the JavaScript code does not do what it is supposed to do.
20. What Is Memoization in JavaScript?
Memoization is a technique that can be used to improve the performance of JavaScript code. Memoization works by storing the results of expensive calculations in a cache. This allows the JavaScript code to avoid re-performing the expensive calculations if the same input is provided again.
For example, the following code calculates the factorial of a number. The factorial of a number is the product of all the positive integers from one to the number.
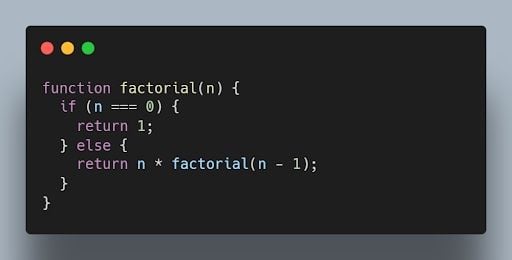
This code can be memoized as follows:
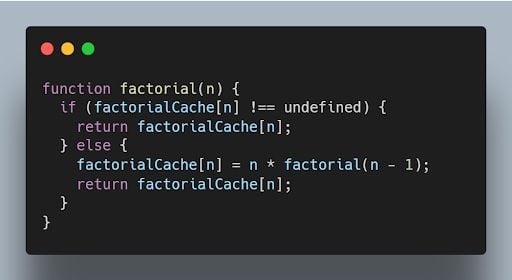
21. What Is Recursion in JavaScript?
Recursion is a programming technique that allows a function to call itself. Recursion can be used to solve a variety of problems, such as finding the factorial of a number or calculating the Fibonacci sequence .
The following code shows how to use recursion to calculate the factorial of a number:
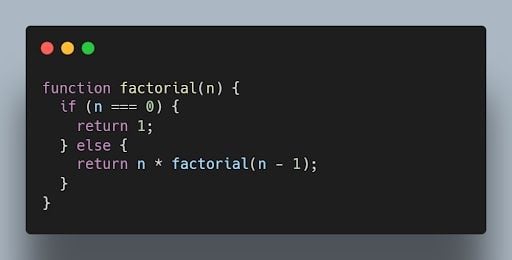
22. What Is the Use of a Constructor Function in JavaScript?
A constructor function is a special type of function that is used to create objects. Constructor functions are used to define the properties and methods of an object.
The following code shows how to create a constructor function:
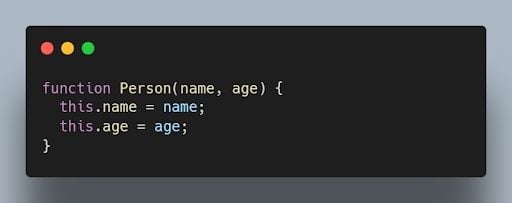
23. What Is the Difference Between a Function Declaration and a Function Expression in JavaScript?
A function declaration is a statement that defines a function. A function expression is an expression that evaluates to a function.
The following code shows an example of a function declaration. This code defines a function named factorial. The factorial function calculates the factorial of a number.
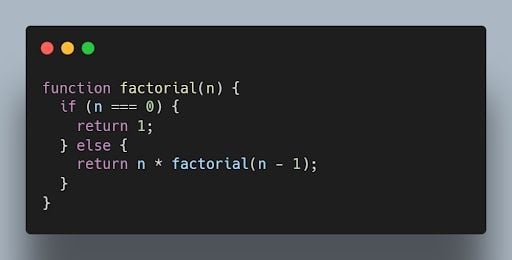
The following code shows an example of a function expression:
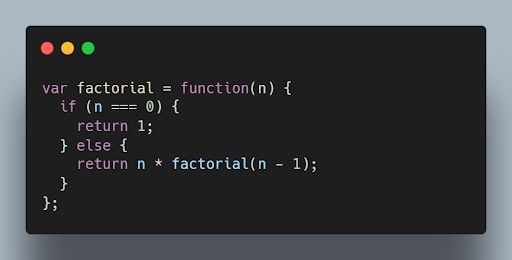
24. What Is a Callback Function in JavaScript?
A callback function is a function passed as an argument to another function, which is then invoked inside the outer function. It allows asynchronous or event-driven programming.
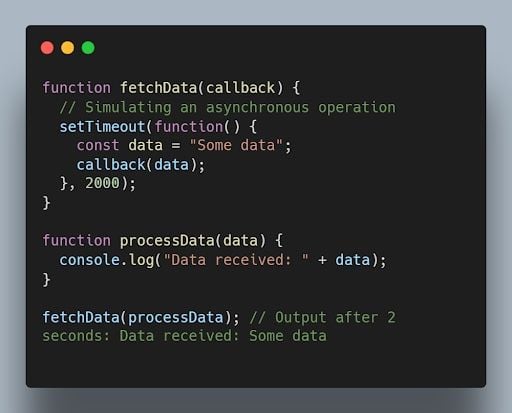
25. What Are Promises in JavaScript?
Promises are objects used for asynchronous operations. They represent the eventual completion or failure of an asynchronous operation and allow chaining and handling of success or error cases.
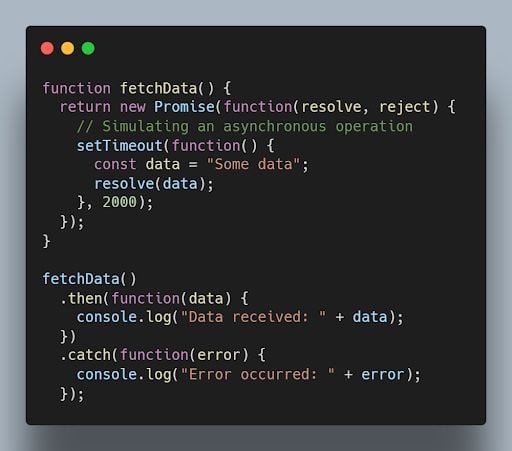
26. What Is the Difference Between Synchronous and Asynchronous Programming?
In synchronous programming, the program execution occurs sequentially, and each statement blocks the execution until it is completed. In asynchronous programming, multiple tasks can be executed concurrently, and the program doesn’t wait for a task to finish before moving to the next one.
Synchronous coding example:
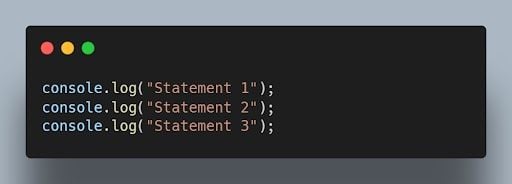
Asynchronous code example:
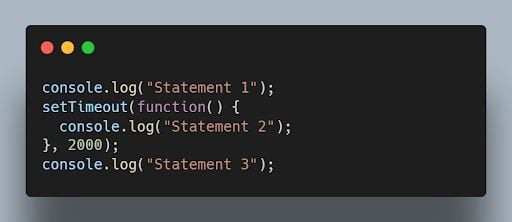
27. How Do You Handle Errors in JavaScript?
Errors in JavaScript can be handled using try - catch blocks. The try block contains the code that may throw an error, and the catch block handles the error and provides an alternative execution path.
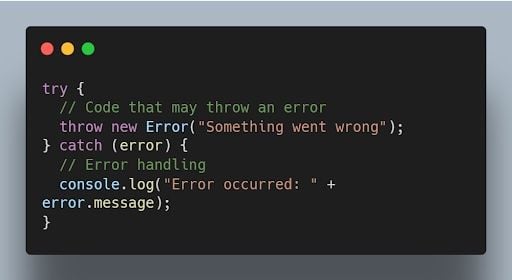
28. Explain the Concept of Event Bubbling in JavaScript.
Event bubbling is the process where an event triggers on a nested element, and then the same event is propagated to its parent elements in the document object model (DOM) tree. It starts from the innermost element and goes up to the document root.
Example:
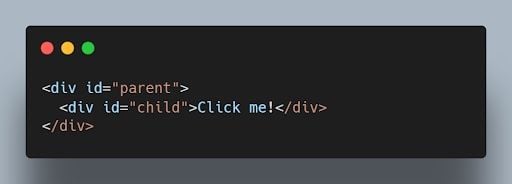
When you click on the child element, both the child and parent event handlers will be triggered, and the output will be:
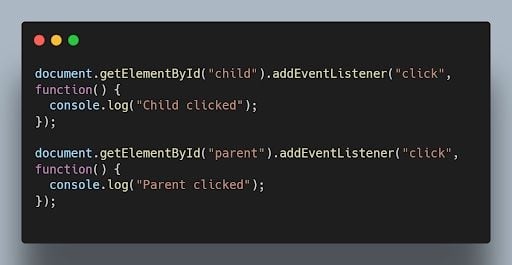
29. What Are Arrow Functions in JavaScript?
Arrow functions are a concise syntax for writing JavaScript functions. They have a more compact syntax compared to traditional function expressions and inherit the this value from their surrounding scope.
For example:
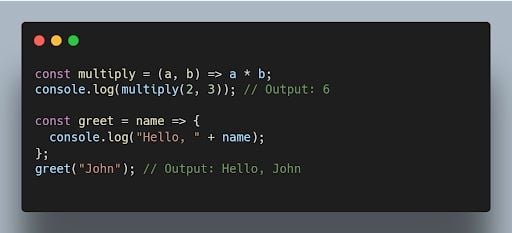
30. What Is the Difference Between querySelector and getElementById?
querySelector is a more versatile method that allows you to select elements using CSS -like selectors, while getElementById specifically selects an element with the specified ID.
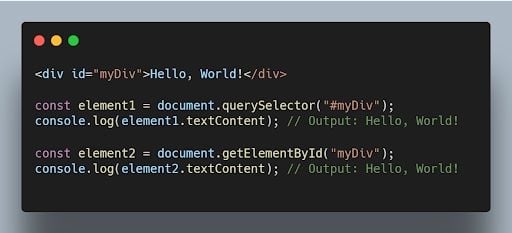
31. What Is the Purpose of the setTimeout() Function in JavaScript?
The setTimeout() function is used to delay the execution of a function or the evaluation of an expression after a specified amount of time in milliseconds.
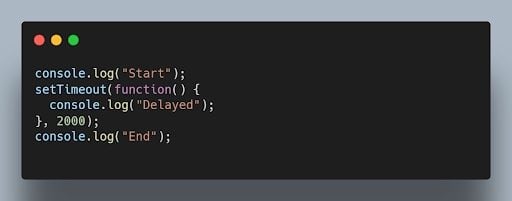
Output after two seconds:
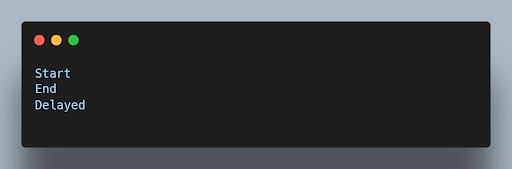
32. What Is Event Delegation and Why Is It Useful?
Event delegation is a technique where you attach a single event listener to a parent element to handle events occurring on its child elements. It’s useful for dynamically created elements or when you have a large number of elements.
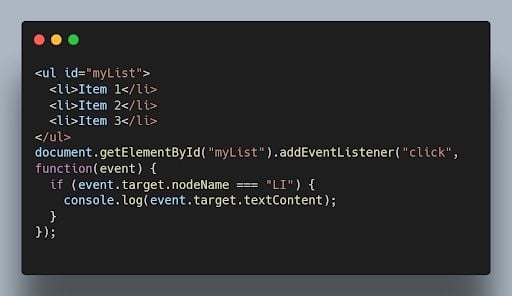
33. How Can You Prevent the Default Behavior of an Event in JavaScript?
You can use the preventDefault() method on the event object within an event handler to prevent the default behavior associated with that event.
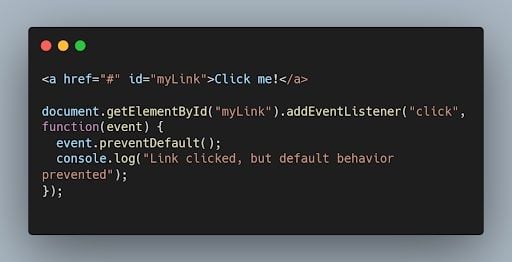
34. What Is the Difference Between localStorage and sessionStorage in JavaScript?
Both localStorage and sessionStorage are web storage objects in JavaScript, but they have different scopes and lifetimes.
- localStorage persists data even after the browser window is closed and is accessible across different browser tabs/windows of the same origin.
- sessionStorage stores data for a single browser session and is accessible only within the same tab or window.
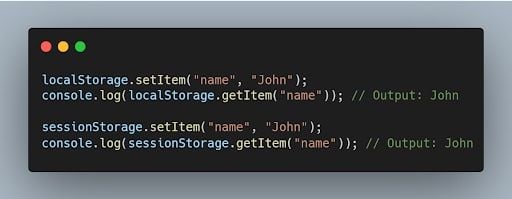
35. How Can You Convert a String to Lowercase in JavaScript?
You can use the toLowerCase() method to convert a string to lowercase in JavaScript.
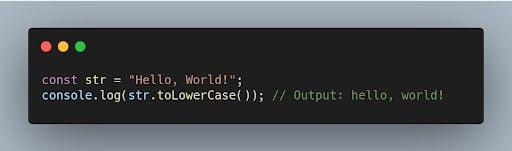
Advanced JavaScript Concepts
36. what is the purpose of the map() function in javascript.
The map() function is used to iterate over an array and apply a transformation or computation on each element. It returns a new array with the results of the transformation.
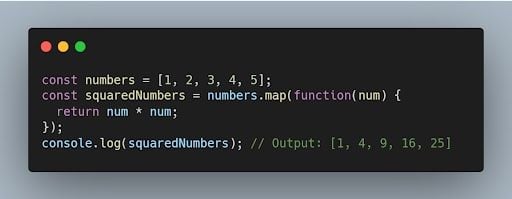
37. What Is the Difference Between splice() and slice()?
- splice() is used to modify an array by adding, removing, or replacing elements at a specific position.
- slice() is used to create a new array that contains a portion of an existing array , specified by the starting and ending indices.
Example of splice() :
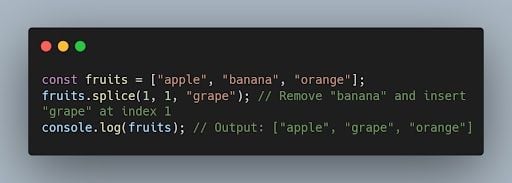
Example of slice() :
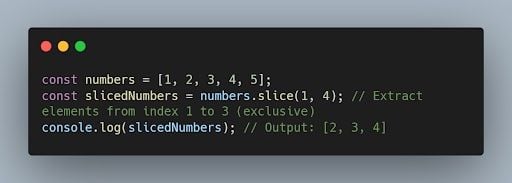
38. What Is the Purpose of the reduce() Function in JavaScript?
The reduce() function is used to reduce an array to a single value by applying a function to each element and accumulating the result.
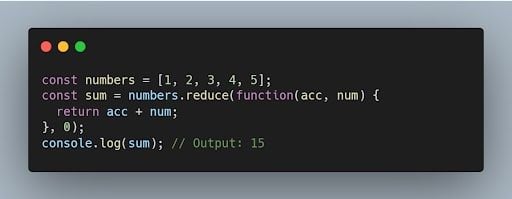
39. How Can You Check if an Array Includes a Certain Value in JavaScript?
You can use the includes() method to check if an array includes a specific value . It returns true if the value is found, and false otherwise.
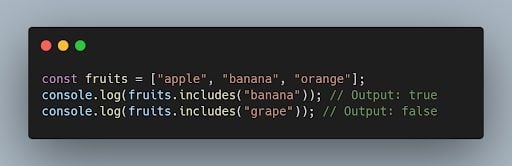
40. What Is the Difference Between Prototype and Instance Properties in JavaScript?
A prototype property is a property that is defined on the prototype object of a constructor function. Instance properties are properties that are defined on individual objects that are created by a constructor function.
Prototype properties are shared by all objects that are created by a constructor function. Instance properties are not shared by other objects.
41. What Is the Difference Between an Array and an Object in JavaScript?
An array is a data structure that can store a collection of values. An object is a data structure that can store a collection of properties.
Arrays are indexed by numbers. Objects are indexed by strings. Arrays can only store primitive data types and objects. Objects can store primitive data types, objects and arrays.
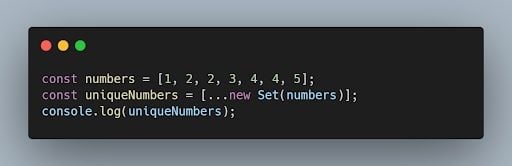
42. How Can You Remove Duplicates From an Array in JavaScript?
One way to remove duplicates from an array is by using the Set object or by using the filter() method with the indexOf() method.
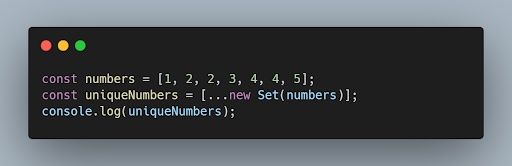
43. What Is the Purpose of the fetch() function in JavaScript?
The fetch() function is used to make asynchronous HTTP requests in JavaScript. It returns a Promise that resolves to the response from the server.
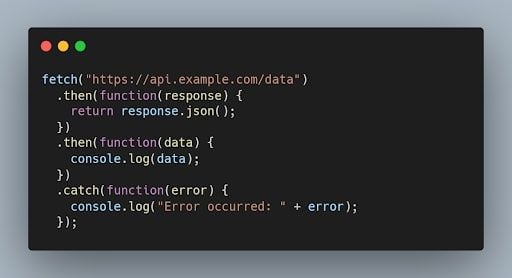
44. What Is a Generator Function in JavaScript?
A generator function is a special type of function that can be paused and resumed during its execution. It allows generating a sequence of values over time, using the yield keyword.
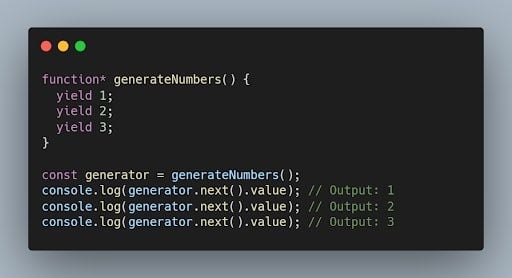
45. What Are the Different Events in JavaScript?
There are many different events in JavaScript, but some of the most common events include:
- Click : The click event occurs when a user clicks on an HTML element.
- Mouseover : The mouseover event occurs when a user's mouse pointer moves over an HTML element.
- Keydown : The keydown event occurs when a user presses a key on the keyboard.
- Keyup : The keyup event occurs when a user releases a key on the keyboard.
- Change : The change event occurs when a user changes the value of an HTML input element.
46. What Are the Different Ways to Access an HTML Element in JavaScript?
There are three main ways to access an HTML element in JavaScript:
- Using the getElementById() method: The getElementById() method takes a string as an argument and returns the HTML element with the specified ID.
- Using the getElementsByTagName() method: The getElementsByTagName() method takes a string as an argument and returns an array of all the HTML elements with the specified tag name.
- Using the querySelector() method : The querySelector() method takes a CSS selector as an argument and returns the first HTML element that matches the selector.
47. What Is the Scope of a Variable in JavaScript?
The scope of a variable in JavaScript is the part of the code where the variable can be accessed. Variables declared with the var keyword have a local scope, which means that they can only be accessed within the block of code where they are declared. Variables declared with the let keyword have a block scope, which means that they can only be accessed within the block of code where they are declared and any nested blocks. Variables declared with the const keyword have a global scope, which means that they can be accessed from anywhere in the code.
48. What Are the Different Ways to Create Objects in JavaScript?
There are multiple ways to create objects in JavaScript, including object literals, constructor functions, the Object.create() method and the class syntax introduced in ECMAScript 2015 (ES6).
Example using object literals:
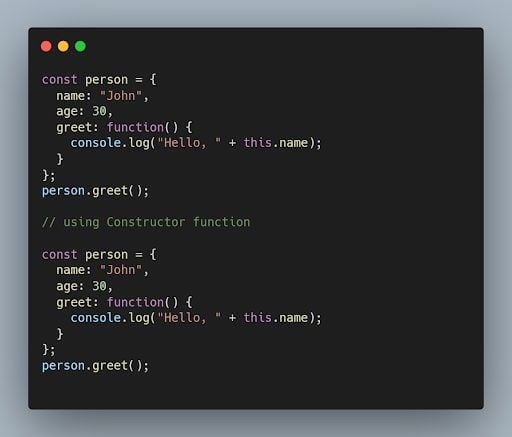
49. What Is the Purpose of the window Object in JavaScript?
The window object represents the browser window. The window object can be used to access the browser’s features, such as the location bar, the status bar and the bookmarks bar.
50. What Is the Purpose of the async and await Keywords in JavaScript?
The async and await keywords are used for handling asynchronous operations in a more synchronous-like manner. The async keyword is used to define an asynchronous function, and the await keyword is used to pause the execution of an async function until a promise is fulfilled or rejected.
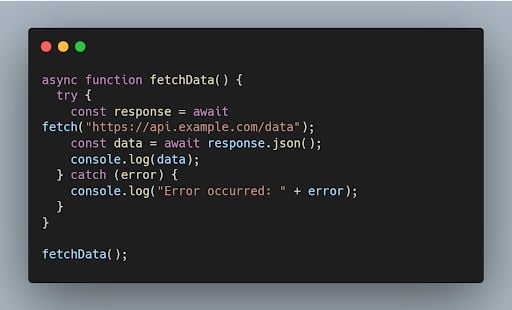
More on JavaScript JavaScript PreventExtensions Method Explained
How to Prepare for a JavaScript Interview
In order to ace a JavaScript interview, you need to be ready for anything. It’s important to practice your code, but you should also be able to clearly explain how different functions work, have real world experience working in JavaScript and understand how to debug.
Fortunately, there are some basic steps you can take to be prepared and stand out from other applicants.
1. Review JavaScript Fundamentals
Make sure you are well-versed in the foundational concepts of JavaScript, such as data types , variables , operators, control structures, functions and objects .
2. Master Key Concepts
It’s also important to study up on key JavaScript topics like promises, asynchronous programming , hoisting, scope, closures, prototypes and ES6 features. You should understand how each of these works.
3. Study Common Interview Topics
Take the time to review JavaScript interview questions that are regularly asked, including those about closures, prototypes, callbacks, promises, AJAX (asynchronous JavaScript and XML), error handling and module systems. Most interviews follow a similar pattern. Preparing answers for those questions will help you stand out from other candidates.
4. Master Debugging Skills
Interviewers for JavaScript will often look to assess your code debugging skills. Practice using IDEs or browser developer tools to find and correct common JavaScript code issues. Learn how to read error messages and review basic debugging techniques.
5. Practice Coding
To develop your coding abilities, complete coding tasks and challenges. Concentrate on standard JavaScript data structures and algorithms such arrays, strings, objects, recursion and sorting techniques.
Online resources like LeetCode , CodeChef and HackerRank can be used to practice coding and get ready for interviews. These websites provide a wide variety of coding puzzles and challenges covering a range of subjects and levels of complexity. They are great resources for developing your coding expertise, problem-solving skills, and algorithmic thinking, all of which are necessary for acing technical interviews.
6. Build Projects
Take on modest JavaScript projects to get experience and show that you can create useful applications. Showing off your portfolio at the interview is also beneficial. In addition, developers can also work on JavaScript projects to obtain practical experience and show that they are capable of building effective applications. A diversified portfolio can be quite helpful when applying for jobs. Platforms like LeetCode, CodeChef, HackerRank and others enable users to develop projects gradually, starting with minor ones and eventually progressing to more ambitious ones.
7. Practice Mock Interviews
With a friend or mentor, practice mock interviews paying particular attention to both behavioral and technical components. This enables you to hear useful criticism and become accustomed to the interview process.
It’s not just mastering the technical aspect of JavaScript, it’s about your body language and how you explain your answers. Many companies are also assessing your ability to work within a team and pair program . The better you can explain your actions and thought process, the more likely you’ll be to win over the interviewer.
Frequently Asked Questions
What are javascript basic interview questions.
Some of the most common basic JavaScript interview questions include:
- What is an array in JavaScript?
- What is closure in JavaScript?
- Why do we use the word “debugger” in JavaScript?
- What's the difference between '==' and '===' In JavaScript?
- What’s the difference between “var,” “let” and “const” in JavaScript?
- What are arrow functions in JavaScript?
How to prepare for a JavaScript technical interview?
To prepare for a JavaScript technical interview, it can be helpful to:
- Review JavaScript fundamentals
- Study and understand common JavaScript concepts (like closures, prototypes, callbacks, promises, error handling)
- Practice JavaScript coding and debugging problems
- Rehearse answers to common interview questions
Are coding interviews hard?
Coding interviews can be difficult because they require a robust understanding of one or more programming languages. These interviews often test for knowledge of algorithms and data structures of a language, and can involve hands-on coding exercises to demonstrate programming abilities.
Recent Career Development Articles
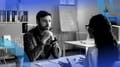
Free Javascript challenges
Learn Javascript online by solving coding exercises.
Javascript for all levels
Solve Javascript tasks from beginner to advanced levels.
Accross various subjects
Select your topic of interest and start practicing.
Start your learning path here
Why jschallenger, a hands-on javascript experience.
JSchallenger provides a variety of JavaScript exercises, including coding tasks, coding challenges, lessons, and quizzes.
Structured learning path
JSchallenger provides a structured learning path that gradually increases in complexity. Build your skills and knowledge at your own pace.
Build a learning streak
JSchallenger saves your learning progress. This feature helps to stay motivated and makes it easy for you to pick up where you left off.
Type and execute code yourself
Type real JavaScript and see the output of your code. JSchallenger provides syntax highlighting for easy understanding.
Join 1.000s of users around the world
Most popular challenges, most failed challenges, what users say about jschallenger.
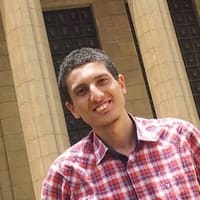
Mohamed Ibrahim
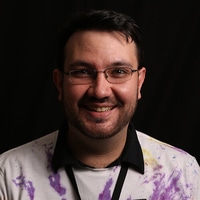
Tobin Shields
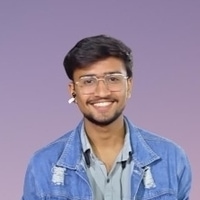
- CSS Animation
- Problem Solving
- JS Interview Question
- UI Components
- Terms & Conditions
- Privacy Policy
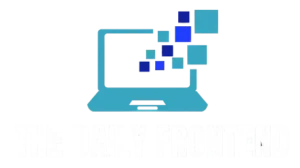
70 Questions to Crack JavaScript Interview Puzzle
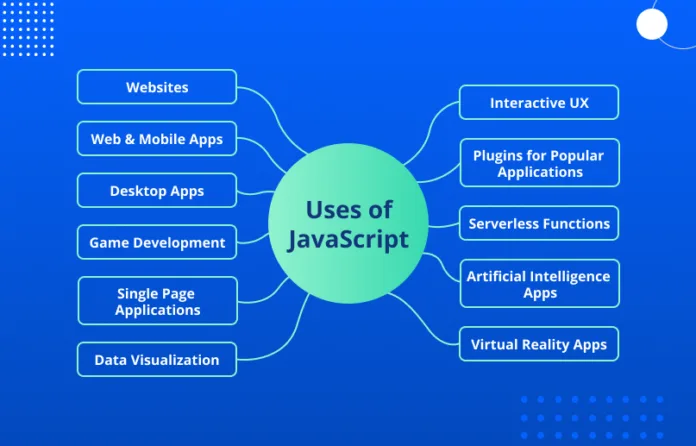
Are you looking to crack your JavaScript interview with ease? Are you tired of searching for relevant and challenging interview questions that can help you nail the interview? Look no further! In this ultimate guide, we’ll present 70 JavaScript interview questions that you must know to become proficient in JavaScript programming.
Essentials of JavaScript
JS interview questions typically test your understanding of basic JS concepts, including variables, datatypes, and operators.
How do you declare and use variables in JavaScript?
- What is the difference between null and undefined?
- What are the primary and secondary datatypes in JS?
Cookies and Sessions
Understand cookies, sessions, and browser local storage.
How to implement cookies in JavaScript? Do you use cookie-exist() function to find existing cookies?
String Functions
JavaScript questions related to string functions such as substring, search, slice, and split might take you back to elementary coding days.
Write an function to reverse a given string in JavaScript. Why don’t we use an iterator for this?
Write an example to search specific substrings in a main string using JavaScript. Any substring can be repeated while looking for in main strings as well as for full phrase.
FString Formatting
JS interview questions around format strings will assess your grip on the syntax. We’ll take you back in time when programming books weren’t just filled with errors.
What are ES5 and ES6 approaches when it comes to sprintf with JavaScript?
Main differences in between `parseInt()` and `FloatParse()` functions?
Advanced object-oriented programming.
Be ready for these advanced OOP, JSON, and asynchronous function execution questions. Interviewer won’t give a hoot about how much "Array.prototype.includes()" matters today.
Can you build one polyfill to check ‘includes’ on "object" as ‘obj.hasOwnProp("a)" using JavaScript?
We might have different browsers while still using ES6 feature includes() ; So write a cross-browser functionality support for "Array.includes()", JavaScript function?
Why a polyfill should add only the new functions defined rather than replace existing library definitions?
Jest and Enzyme in React
JS interview questions targeting popular libraries and frameworks – for instance, React (also known as JSX by your interviewer).
Why testing component with jest does provide data to components using JSX files?
Write unit-tests as well as test if these components correctly receive proper functions and functions within tests correctly.
JavaScript interview questions targeting important functions and ES6 Syntax that are the standard use as well. In regards “async” “as Promise” & ` async = await ` which, by the way, gives control to an action but this is “the problem”: it takes “only some” steps but they run in “different positions than the one given code runs”. Please help them if you can.
How is Node using by JavaScript and which ‘API’ will make its API?
There are other more crucial reasons for choosing an online JSON API using JSON schema but the one above with one of its examples like here. ## Conclusion Cracking a JavaScript interview requires a solid understanding of fundamental JavaScript concepts, including variables, functions, and control statements, as well as your coding skills, syntaxes with error handling. So do not let JavaScript keep throwing you a curve at once. Take it out today and see for best preparation.
RELATED ARTICLES MORE FROM AUTHOR
Solving complex problems with javascript recursion examples, mastering javascript coding problem-solving with practice and patience, boosting your coding skills with javascript algorithm problem-solving techniques, leave a reply cancel reply, latest posts, javascript this keyword: tips and tricks, designing effective ui components for settings pages, responsive html images: tips for smaller file sizes and faster load..., mastering css animation in html for web developers, editor picks, popular posts, best way to learn css, make div to fill the empty space using css, html iframe usage: a comprehensive guide, popular category.
- CSS Animation 493
- Javascript 321
- Problem Solving 276
- JS Interview Question 233
- UI Components 191
- Frameworks and Libraries 155
- Web Performance Optimization 147

IMAGES
COMMENTS
52 Node.js Interview Questions (ANSWERED) for JavaScript Developers As an asynchronous event-driven JavaScript runtime, Node.js is designed to build scalable network applications. Follow along to refresh your knowledge and explore the 52 most frequently asked and advanced Node JS Interview Questions and Answers every...
CodeChef offers a catalog of JavaScript problems for beginners to practice and prepare for interviews. The problems cover topics such as output, variables, strings, user inputs, conditional statements, loops, arrays, functions, and algorithms.
5 days ago · JavaScript (JS) is the most popular lightweight, scripting, and interpreted programming language. It was developed by Brendan Eich in 1995. JavaScript is well-known as a scripting language for web pages, mobile apps, web servers, and many other platforms. It is essential for both front-end and back-
Prepare for your JavaScript interview with these 50 coding questions and solutions. Learn basic and advanced concepts, algorithms, and techniques to impress your hiring manager.
Oct 30, 2024 · JavaScript Interview Questions and Answers With Examples. JavaScript interview questions range from the basics like explaining the different data types in JavaScript to more complicated concepts like generator functions and async and await. Each question will have answers and examples you can use to prepare for your own interview.
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies.
Practice JavaScript coding with fun, bite-sized exercises. Earn XP, unlock achievements and level up. It's like Duolingo for learning to code.
JSchallenger. Free Javascript challenges. Learn Javascript online by solving coding exercises. Javascript for all levels. Solve Javascript tasks from beginner to advanced levels.
Sep 30, 2024 · In this comprehensive guide, we’ll share over 70 JavaScript problem-solving exercises to help you build a strong foundation in programming and become a more effective developer. Why Problem-Solving Exercises are Essential for JavaScript Developers. Problem-solving exercises are an essential part of any developer’s training.
Oct 2, 2024 · JavaScript interview questions targeting important functions and ES6 Syntax that are the standard use as well. In regards “async” “as Promise” & ` async = await ` which, by the way, gives control to an action but this is “the problem”: it takes “only some” steps but they run in “different positions than the one given code runs”.